Final Review-Fall 2007
Below are questions like those I plan to ask on the Final Exam (December 10 2:50-5:40).
Please do try these questions! Post your answers, questions, comments, concerns, and criticisms on the pages for each question. Those comments, questions, etc. can also be about each others' answers! If someone posts an answer that you don't understand, ask about it! If you see a question here that you know the answer to, don't keep it to yourself – help your fellow students!
I will be reading your answers. Here are the ground rules for the interaction.
- If you don't post, neither will I. I will not be posting official solutions to these problems at all! If one of you gets it right, terrific!
- I will try to always point out if an answer is wrong. I won't always point out when the answer is right.
- I am glad to work with you toward the right answer. I will give hints, and I'm glad to respond to partial guesses.
Definition Time (Sorry this is a data structures course after all)
Data Structures
Graph
Tree
Binary Trees
Linked List
Circular Linked List
Array
Queue
Stack
Questions, comments, answers at Final exam review Fall2007:Definition Time
Turtle Initial
Below is the example turtle drawing from class. Create a TurtleInitial class with a main() method that draws the first initial of your family (last) name.
public class MyTurtlePicture {
public static void main(String [] args) {
//FileChooser.setMediaPath("C:/cs1316/MediaSources/");
Picture canvas = new Picture(600,600);
Turtle jenny = new Turtle(canvas);
Picture lilTurtle = new Picture(FileChooser.getMediaPath("Turtle.jpg"));
for (int i=0; i <=40; i++){
if (i < 20)
jenny.turn(20);
else
jenny.turn(-20);
jenny.forward(40);
jenny.drop(lilTurtle.scale(0.5));
}
canvas.show();
}
}
Questions, comments, answers at Final exam review Fall2007:Turtle Initial
Writing methods on the Picture class
The following is the clearBlue() method of the class Picture.
/*** Method to clear the blue from the picture (set
*the blue to 0 for all pixels*/
public void clearBlue()
{
Pixel[] pixels = this.getPixels();
Pixel pixel = null;
int index = 0;
while(index < pixels.length)
{
pixel = pixels[index];
pixel.setBlue(0);
index++;
}
}
Write a method to draw vertical stripes (leaving a gap for the original picture between every stripe) down the picture. Have every-other stripe be red, and the others be green.
Public void vertical_stripes()
{
}
Questions, comments, answers at Final exam review Fall2007:Writing methods on the Picture class
Sound Manipulation
Complete the method definition below that reverses an instance of Sound.
/**
* Method to reverse a sound.
**/
public Sound reverse( ){
Sound target = new Sound(getLength());
int sampleValue;
}
Questions, comments, answers at Final exam review Fall2007:Sound Manipulation
Draw the Tree
Below is a method from SoundTreeExample. Draw the data structure that it defines. BE SURE TO LABEL children versus next nodes!
public void setUp2() {
FileChooser.setMediaPath("D:/cs1316/MediaSources/");
Sound clap = new Sound(FileChooser.getMediaPath("clap-q.wav"));
Sound chirp = new Sound(FileChooser.getMediaPath("chirp-2.wav"));
Sound rest = new Sound(FileChooser.getMediaPath("rest-1.wav"));
Sound snap = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
Sound clink = new Sound(FileChooser.getMediaPath("clink-tenth.wav"));
Sound clave = new Sound(FileChooser.getMediaPath("clave-twentieth.wav"));
Sound gong = new Sound(FileChooser.getMediaPath("gongb-2.wav"));
Sound guzdial = new Sound(FileChooser.getMediaPath("guzdial.wav"));
Sound is = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundBranch();
SoundNode sn;
SoundBranch branch1 = new SoundBranch();
sn = new SoundNode(guzdial.append(is).append(snap));
branch1.addChild(sn);
sn = new SoundNode(clink.append(snap).append(clave));
branch1.addChild(sn);
sn = new SoundNode(guzdial.append(is).append(is));
branch1.addChild(sn);
root.addChild(branch1);
scaledBranch = new ScaleBranch(2.0);
sn = new SoundNode(clink.append(clave).append(gong));
scaledBranch.addChild(sn);
sn = new SoundNode(rest.append(chirp).append(clap));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
scaledBranch = new ScaleBranch(0.5);
sn = new SoundNode(guzdial.append(is).append(gong));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
SoundBranch branch2 = new SoundBranch();
sn = new SoundNode(clap.append(clap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
root.addChild(branch2);
root.playFromMeOn();
}
Questions, comments, and answers at Final exam review Fall2007:Draw the Tree
Inheritance
public abstract class Car {
private double mpg;
public abstract void drive();
public void repair() { System.out.println("Car’s repair"); }
}//end class Car
public class Toyota extends Car {
public void drive() { System.out.println("Toyota’s drive"); }
}//end class Toyota
public class Avalon extends Car {
public void drive() { System.out.println("Avalon's drive"); }
public void zoom() { System.out.println("Avalon's zoom"); }
}//end class Avalon
public class Camry extends Toyota {
public void drive() { System.out.println("Camry's drive"); }
public void washMe() { System.out.println("Camry's washMe");}
}//end class Camry
Given the above classes decide whether the following segments of code will compile or not (be sure to consider both lines):
Car a = new Camry();
a.repair();
Avalon b = new Car();
b.drive();
Car c = new Car();
c.drive();
Car d = new Camry();
d.drive();
Toyota e = new Toyota();
e.repair();
Car f = new Avalon();
f.zoom();
Car g = new Avalon();
((Avalon)g).zoom();
Car h = new Camry();
((Avalon)h).zoom();
Given
Toyota i = new Camry();
i.drive();
What is the output?
Questions, comments, and answers at Final exam review Fall2007:Inheritance
Writing a Linked List Method
Write an insertBefore method that will take two LLNode’s (target and newNode) and insert newNode before target. So if a list is set up as A->B->C->D->E->null and we call
A.insertBefore(D, X);
Then the new list will look like A->B->C->X->D->E->null
You can assume you are in the LLNode class. If you need extra room use the previous page. Use comments to help make your code understandable.
Questions, comments, answers at Student894
Compare the GUIs
A. Draw the tree described by the below two classes.
B. Explain the difference in what the user sees from creating instances of these two classes. Relate it to trees and rendering.
EXAMPLE ONE:
/**
* A GUI that has various components in it, to demonstrate
* UI components and layout managers (rendering)
**/
import javax.swing.*; // Need this to reach Swing components
import java.awt.*; // Need this to reach FlowLayout
public class GUItreeFlowed extends JFrame {
public GUItreeFlowed(){
super("GUI Tree Flowed Example");
this.getContentPane().setLayout(new FlowLayout());
/* Put in a panel with a label in it */
JPanel panel1 = new JPanel();
this.getContentPane().add(panel1);
JLabel label = new JLabel("This is panel 1!");
panel1.add(label);
/* Put in another panel with two buttons in it */
JPanel panel2 = new JPanel();
this.getContentPane().add(panel2);
JButton button1 = new JButton("Make a sound");
panel2.add(button1);
JButton button2 = new JButton("Make a picture");
panel2.add(button2);
this.pack();
this.setVisible(true);
}
EXAMPLE TWO:
/**
* A GUI that has various components in it, to demonstrate
* UI components and layout managers (rendering)
**/
import javax.swing.*; // Need this to reach Swing components
import java.awt.*; // Need this to reach BorderLayout
public class GUItreeBordered extends JFrame {
public GUItreeBordered(){
super("GUI Tree Bordered Example");
this.getContentPane().setLayout(new BorderLayout());
/* Put in a panel with a label in it */
JPanel panel1 = new JPanel();
this.getContentPane().add(panel1,BorderLayout.NORTH);
JLabel label = new JLabel("This is panel 1!");
panel1.add(label);
/* Put in another panel with two buttons in it */
JPanel panel2 = new JPanel();
this.getContentPane().add(panel2,BorderLayout.SOUTH);
JButton button1 = new JButton("Make a sound");
panel2.add(button1);
JButton button2 = new JButton("Make a picture");
panel2.add(button2);
this.pack();
this.setVisible(true);
}
}
Questions, comments, and answers at Final exam review Fall2007:Compare the GUIs
Understanding UML
Answer the questions based on the following UML diagram. Note: the labels for the association between Flight and CrewMember are reversed.
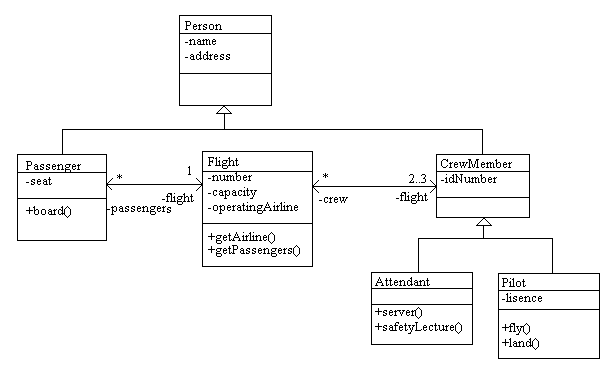
A. List all of the methods in an instance of Attendant.
B. How does a Pilot access all of the passengers on the flight?
C. List all of the classes that understand name.
D. List all of the instance variables in an instance of Flight.
E. By what names does a flight know all of its crew members?
F. How many crew members are there on every flight?
Questions, comments, answers at Final exam review Fall2007:Understanding UML
Slow! Sick People Crossing!
Below is the code for the class PersonAgent from the DiseaseSimulation example. REMOVEDge it so that, when people get sick, they slow down by 75%.
import java.awt.Color; // Color for colorizing
import java.util.LinkedList;
/**
* PersonAgent -- Person as a subclass of Agent
**/
public class PersonAgent extends Agent {
public boolean infection;
/**
* Initialize, by setting color and making move fast
**/
public void init(Simulation thisSim){
// Do the normal initializations
super.init(thisSim);
// Make it lightGray
setColor(Color.lightGray);
// Don't need to see the trail
setPenDown(false);
// Start out uninfected
infection = false;
// Make the speed large
speed = 100;
}
/**
* Count infected
**/
public int infected() {
int count = 0;
LinkedList agents = simulation.getAgents();
PersonAgent check;
for (int i = 0; i<agents.size(); i++){
check = (PersonAgent) agents.get(i);
if (check.infection) {count++;}
}
return count;
}
/**
* Become infected
**/
public void infect(){
this.infection = true;
this.setColor(Color.red);
// Print out count of number infected
System.out.println("Number infected: "+infected());
}
/**
* How a Person acts
**/
public void act()
{
// Is there a person within infection range of me?
PersonAgent closePerson = (PersonAgent) getClosest(20,
simulation.getAgents());
if (closePerson != null) {
// If this person is infected, and I'm not infected
if (closePerson.infection && !this.infection) {
// I become infected
this.infect();
}
}
// Run the normal act() -- wander aimlessly
super.act();
}
////////////////////////////// Constructors ////////////////////////
// Copy this section AS-IS into subclasses, but rename Agent to
// Your class.
/**
* Constructor that takes the model display (the original
* position will be randomly assigned)
* @param modelDisplayer thing that displays the model
* @param thisSim my simulation
*/
public PersonAgent (ModelDisplay modelDisplayer,Simulation thisSim)
{
super(randNumGen.nextInt(modelDisplayer.getWidth()),
randNumGen.nextInt(modelDisplayer.getHeight()),
modelDisplayer, thisSim);
}
/** Constructor that takes the x and y and a model
* display to draw it on
* @param x the starting x position
* @param y the starting y position
* @param modelDisplayer the thing that displays the model
* @param thisSim my simulation
*/
public PersonAgent (int x, int y, ModelDisplay modelDisplayer,
Simulation thisSim)
{
// let the parent constructor handle it
super(x,y,modelDisplayer,thisSim);
}
}
Questions, comments, and answers at Final exam review Fall2007:Slow! Sick People Crossing!
Short Answer
What is the difference between a Discrete Simulation and a Continuous Simulation?
How is the Event Queue used in a Discrete Simulation?
What is meant by a method being declared to be static?
Often we see references to variables that are in all caps. An example would be SOUTH in the BorderLayout class. What does this typically mean?
What is the difference between extends and implements?
What is the point of UML?
What are the different types of relationships between classes and how are they designated in UML?
What is the advantage to a binary search tree ( think "big oh" O() )? What is the perfect Binary Search Tree?
We used random number generators to help control the execution of continuous and discrete event simulations. How were they used and why? What kinds of distributions did we discuss and how are they distinguished?
Questions, comments, answers at Final exam review Fall2007:Short Answer
Links to this Page