Homeworks-Spring 2008
Homework 0: Setup [Questions]
Homework 1: AdvancedPicture Methods [Questions] [Gallery]
Homework 2: Drawing Initials with LetterTurtle [Questions] [Gallery]
Homework 3: Writing a Linked List [Questions]
Homework 4: You be the Composer [Questions]
Homework 5: Family Tree [Questions]
Homework 6.1: Train Your Turtle to Draw on Command [Questions]
Homework 6.2: Turtle Etch-A-Sketch [Questions]
Homework 6.3: The Demo [Questions]
Homework 7: Enhancing Simulations [Questions]
Homework 8: [Questions]
Bonus Homeworks
Bonus Homework 1: TurtleDance [Questions][Gallery]
Bonus Mini Homework 1: TurtleCommands [Questions]
Bonus Mini Homework 2: Fix the Buggy Code!
Bonus Mini Homework 3: Explain from the Student’s Perspective [Questions]
Bonus Mini Homework 4: AbstractContactList [Questions]
DUE DATE: Wednesday January 16, 7pm (not for credit)
Back to the top
DOWNLOAD AND SET UP DR. JAVA USING THE INSTRUCTIONS
GETTING USED TO THE INTERACTIONS PANE
One of the appeals of Dr. Java is the INTERACTION PANE, which can be used to run simple Java commands without setting up a whole class. The INTERACTIONS PANE is the bottom pane in Dr. Java’s interface.
Type the following line in the INTERACTIONS PANE:
System.out.println("Hello World.");
and press enter. System.out.println(...) is used to output to the terminal and is very useful for simple debugging.
Now try creating two integers:
int a = 6;
int b = 3;
You can add the two integers together:
int sum = a + b;
You can also multiply them:
int product = a *b;
subtract them:
int difference = a – b;
divide them:
int quotient = a / b;
and many other things as well.
Now maybe it would be best to print out each result, but it would be hard to discern one number from the other if we just printed the numbers (as Strings) without distinguishing them in any way. This notion will be especially important when you have several printouts within a program that will print out in different orders or even not at all. String concatenation is where you combine two or more Strings together. To concatenate two Strings in Java you use the "+" operator. Thus if we had the following:
String s1 = "Georgia";
String s2 = "Tech";
String space = " ";
We can create the String "Georgia Tech" by concatenating s1, s2 and space together.
System.out.println(s1 + space + s2);
Now do some more String manipulations:
String myName = "Dawn";
System.out.println("Hi, my name is " + myName);
System.out.println("I have two numbers " + a + " and " + b);
System.out.println("The sum is " + sum);
System.out.println("The product is " + product);
System.out.println("The difference is " + difference);
System.out.println("The quotient is " + quotient);
CHECKING IF EVERYTHING IS SET UP CORRECTLY
Open up FileChooser.java and Picture.java in the java-sources folder we provided to you. Compile those two files using the compile button found in the upper right hand area (Ignore any warnings).
Let us go ahead and set your media path (Keep in mind that C:/CS1316/mediasources/ is the media path and may be different on your computer. Please adjust as needed).
FileChooser.setMediaPath("C:/CS1316/mediasources/");
To make sure it worked, try loading a picture:
Picture p = new Picture(FileChooser.getMediaPath("swan.jpg"));
p.show();
Setting up your media path means that the program will store the path to your media sources folder inside of a file and retrieve it each time you need to use it. So instead of hardcoding C:/CS1316/mediasources/swan.jpg each time, you use FileChooser.getMediaPath("swan.jpg") which retrieves the media path, C:/CS1316/mediasources/, and concatenates it with swan.jpg to create C:/CS1316/mediasources/swan.jpg. This is necessary for your assignments, because media paths will differ from computer to computer.
CREATING YOUR FIRST CLASS
In Dr. Java make a new file and save it as HW0.java. Now inside HW0.java you will define your first class and your first main method:
public class HW0 {
public static void main(String [] args){
//This is my first comment
System.out.println("I have just written my first class!");
}
}
You will have no problem understanding the code above a little later on in the course. For now go ahead and save the code and compile it using the compile button.
Now run the code using the interactions pane:
java HW0
You should get an output of I have just written my first class!
Now try to write some comments. Above public class HW0 write your name, prism account (same as your gatech email) and your recitation section. You can write it in one of two ways:
//Dawn Finney
//gth683e
//A2
OR
/*Dawn Finney
gth683e
A2*/
/**/ are for multi-line comments while // are for single-line.
WHAT TO TURN IN
HOW TO TURN IT IN
Ask Questions on HW0-Spring08 here.
Back to the top
DUE DATE: Friday January 18, 7pm
Back to the top
For this assignment, modify the AdvancedPicture.java class that extends Picture and write two new methods in AdvancedPicture that implement two different kinds of image manipulation.
Image Manipulation
The type of image manipulation implemented is entirely up to you. However, you must implement a manipulation that does not already exist within the Picture class. You are more than welcome to use the methods within Picture as a guide, but we expect you to write your own methods and to not make any calls to existing Picture methods.
Self-Documentation
Suppose we have two methods named intensifyColors() and method1(). Can you guess as to what each method will do without testing the method or reading through the code? intensifyColors() seems like it will make the colors brighter or sharper somehow, while method1() could do anything. Now imagine a giant program where all the methods were named similar to method1(). Get the big picture now? Your methods should really have names that reflect the manipulations that will occur. Giving your methods names that describes what happens within the method is called self- documentation. Self-documentation makes it easier for others to understand what the method is supposed to do and the grand scheme of things what your program will do.
Commenting
Though your method names are self-documenting you should still comment your code. Place above each of your method names, comments about what the method will do. Also always place comments that include your name, prism ID (gtx000x or jsmith3) and grading TA at the top of all the java files you submit. Some people when asked to comment their code will do so after all of the code is already written and working. While this is an acceptable way of commenting, some people find it easier to write an outline of how you want to approach a program using comments. Essentially we use write a program to achieve some goal or complete some task. While some people can start hacking their way into some semi-functioning code without much forethought, it is highly recommended that you actually sit and think about the steps you should take. Regardless of when you comment, you should comment and will be required to comment in this homework and forthcoming homeworks.
Looping
Also you will be required to use a for loop in one method and a while loop in the other. The method you write will be called on the picture that needs to be manipulated. For instance, after this sequence of code:
AdvancePicture ap = new AdvancedPicture(FileChooser.getMediaPath("swan.jpg "));
ap.intensifyColors();
ap.show();
you would expect ap to be manipulated in some way.
What to Return from the Methods
You are only required to write void methods, methods that do not return anything after it is preformed. If you so desire, you may write non-void methods, methods that does return something after completion, but know that it is not required for this assignment.
Testing the Methods
Remember that you were first introduced to the main method in homework 0 where your HW0 class contained a public static void main(String[]args). You should also include a main method within AdvancedPicture to test your new picture manipulation methods. Remember to test both methods preferably on a fresh picture each time.
You may not include FileChooser.setMediaPath() anywhere in your code. You must use FileChooser.getMediaPath() and may not hardcode any picture paths in your homework.
What to Turn In
- AdvancedPicture.java (Be sure to submit the .java file and not the .java~ or .class!)
How to Turn In
Did you make an interesting picture with your first methods? Please consider sharing it in the Gallery HW1: AdvancedPicture methods-Spring08
Ask Questions on HW1-Spring08 here.
Back to the top
DUE DATE: Friday January 25, 7pm
Back to the top
Provided Files: LetterTurtle.java
For this assignment, you will be required to draw the first letters of your first and last name using a LetterTurtle.
The Initials
You will be expected to draw the first letters of your first and last name, which will henceforth be referred to as your initials for the purposes of this homework. For me, Dawn Finney, this means that I should draw the letters “DF” to receive credit. If your initials are comprised of the same letters, then you must choose another letter to do, though which letter is entirely up to you.
The Shape of the Letters
We realize the difficulty of creating letters that have curves such as D, O, S, B to name a few. Those with initials that have curves may draw the “block” or “square” versions of the curved letters instead. Those wishing to attempt curved letters are welcome to do so for extra credit. Please see the extra credit section.
Extra Credit (+5 for each extra method)
In addition to the block form of the letters, you can choose 1 or 2 more letters and write methods for the curved version. If there is no curved version of your initials, feel free to choose any curved letter to do. Just drawing the curved versions of your initials will also satisfy the minimum requirements, but you will not receive extra credit. Thus for my initials “DF,” I would have to write one method for the block version of “D” and a method for “F” to satisfy the minimum requirements. To receive extra credit, I could write a method for the curved version of “D” and chose another letter since there is no curved version of “F.”
Method Header
You will need to write at least a separate method for each letter. The method names again, like homework 1, need to be appropriate so as to be self-documenting. The methods will take in a starting integer x coordinate and starting y coordinate. How the starting coordinate will correspond to your letter is up to you, but will need to be detailed in your comments. Again the minimum requirement will be for both methods to be void, but non-void method will be accepted as well.
For example, the methods I would have to write would be something akin to the following:
public void drawD(int x, int y) {
//Method body in here
}
public void drawF(int x, int y){
//Method body in here
}
Method Body
You will be required to do your drawing with the LetterTurtle on a World. To make the drawing easier to see, you need to change the Pen width of the LetterTurtle to something other than the default width of 1. The final resulting drawing cannot contain a visible LetterTurtle.
Useful Mathematical Values and Methods
When drawing your initials especially the curved ones, you will probably need some typical mathematical values and methods. You will find these useful values and methods within the Math class in the Java API:
http://java.sun.com/j2se/1.5.0/docs/api/java/lang/Math.html
For instance if I wanted to use the cos method and constant value PI, I could do something like the following:
double value = Math.cos(Math.PI * 0);
Casting
Because a great deal of the LetterTurtle movement methods require int values as inputs and most Math methods return double values, it is necessary to cast. In general, one of the java compiler’s tasks is to make sure that data types match across an assignment statement and between the formal and actual parameters of a method, and to show a compilation error if these are not consistent.
When we cast a value to a different data type, we inform the compiler that we really do know what we are doing, and that it should not worry about type consistency. Specifically, if we cast a double to type int, we are promising two things to the compiler:
1. We believe the contents of that double result to be small enough to fit in an int, and
2. We are happy to discard the fractional part of the double that is not stored in an int.
Here is the same line of code previously given:
double value = Math.cos(Math.PI * 0);
What if value was actually an int like so:
int value = Math.cos(Math.PI * 0);
Just looking at the code, you know you would get an error, because the types on either side of equal sign do not match. Currently we have:
int = double
We need the same type on both sides to get past the compiler. Because we need int for our LetterTurtle method, we are going to cast the right side of the equal sign to int:
int value = (int)(Math.cos(Math.PI * 0));
Here we finally have the same type on both sides:
int = int
It is important to note that to change a double to an int, the double is truncated at the decimal point. Essentially this means the decimal and everything to the right of it is thrown away. It is very important to note that does casting will not round the value up or down.
Examples (done in Dr. Java’s interactions pane):
> (int)5.123
5
> (int)6.523
6
> (int)-9.791
-9
Commenting
Though your method names will be self-documenting you should still comment your code. Place above each of your method names, comments about what the method will do. Also always place comments that include your name, prism ID (gtx000x or jsmith3) and grading TA at the top of all the java files you submit.
Main Method
You should also include a main method within LetterTurtle to test your drawing methods.
Grading REMOVEDges
Several grading changes will be in place for homework 2 as the penalties for not following directions increase. Below are the grading rules from homework 2 on:
- Homeworks that do not compile or contain hardcoded mediapaths will result in an automatic zero.
- Homeworks that do not include all necessary files to run and all files required by the assignment will result in an automatic zero.
- Homeworks that include FileChooser.setMediaPath() will result in an automatic letter drop (-10pts).
- Homework assignment submitted via email will not be accepted.
What to Turn In
How to Turn In
Ask Questions on HW2-Spring08 here.
Back to the top
DUE DATE: Friday February 15, 7pm
Back to the top
Provided Files: HW3javadoc-4.rar
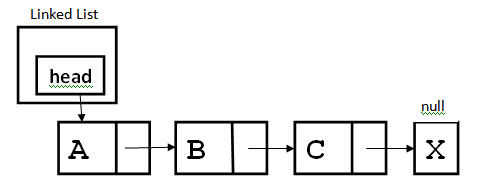
A linked list is a dynamic data structure that stores information through nodes. Each node is a data structure itself that stores information and the next node that it references. Unlike an array, a linked list can be adjusted without moving any data over to a new array or without shifting over any information. Therefore, it can be beneficial in many ways depending on the task at hand.
As a linked list, you only know about the first node. That node has its data element and then points you to the next node. To find the last element in the list you need to start at the first node and traverse the list node by node until you run out of elements (if the next of a node points to null, then you are at the end of the list)
For Homework 3 you will be creating a linked list that stores contact information for your friends. You will be turning in 3 files: ContactList.java, ContactNode.java, ContactEntry.java.
ContactEntry.java
Since we will be making a linked list of contacts, it makes sense to write an Object that can hold information required for a friend. You will be a simple address book entry, but you still need three data fields to store name, phone number, and email address. These data fields must be private and you will use accessors and modifiers to view and modify them. You will also need constructors to initialize your ContactEntry. You will need the following constructors:
public ContactEntry(){..}
public ContactEntry(String name, String phone, String email){..}
You may have more constructors then that, but those are the required elements. You will also need a toString() method which returns a String summarizing the information about your contact.
ContactNode.java
This is your node that will make up your linked list. It needs to have a data element (of type ContactEntry) and it will need to have a data element that will be the next node in the list (of type ContactNode). These elements also have to be private and have the appropriate accessors and modifiers. You will be required to have a constructor, public ContactNode(ContactEntry data), that creates a stand alone node with data as its data element. You will also be required to have a toString() that summarizes the information in your data element (the contact).
ContactList.java
This is the actual linked list and will be your entry point into your address book. It will not have access to every element, just the first one (typically called head). Once again your head data element will be private and will need to include the appropriate accessors and modifiers. This is where you will insert elements and remove elements from your address book. Be sure to include a constructor that initializes the list by setting head to null. It will also need to have the following methods:
public ContactList(){…}
public ContactList(ContactNode head){…}
public ContactNode getHead(){…}
public void setHead(ContactNode head){…}
public void add(ContactNode contactToInsert){…}
public boolean insertAfter(ContactNode node, ContactNode contactToInsert){…}
public boolean delete(ContactNode node){…}
public ContactEntry searchByName(String name){…}
public ContactEntry searchByPhone(String phone){…}
public ContactEntry searchByEmail(String email){…}
public int size(){…}
public String toString(){…}
Bonus (+10pts)
Allow your users to sort your list. Add methods sortByName(), sortByPhone(), and sortByEmail() to your ContactList.
What to Turn In
- ContactEntry.java
- ContactNode.java
- ContactList.java
How to Turn In
Ask Questions on HW3-Spring08 here.
Back to the top
DUE DATE: Saturday February 23, 7pm
Back to the top
Provided Files: HW4javadocs.rar, AdvancedSongList.java, AdvancedSongNode.java
For homework 4, you will be selecting a song of your choice (see below for some ideas). You will need to know the notes in the song that you choose. If you cannot or do not want to compose the music yourself, you should start by selecting sheet music for a song that piques your interest. Free sheet music can be found all over the internet (Google is your friend) but for two references we will give you are: http://www.musicnotes.com/ and http://freesheetmusic.net/.
Note: We are expecting music and not a random cacophony of notes. You will not receive full credit if you do not turn in music.
Music Theory 101
First you will need to create a class called AdvancedSongPhrase that will extend SongPhrase and only have the necessary riffs for your song. See the SongPhrase class for working examples. You must create at least four new riffs in this class and each riff must contain at least two measures or 8 notes.
Next you will need to create a new class (of some name of your choosing) with a main that will assemble your song and then open your song with showFromMeOn (or whatever you need to do open up the notation View on your masterpiece). Your riffs will be placed into AdvancedSongNodes which will then be assembled into a linked list, AdvancedSongList. You are required to use either repeatNext or repeatNextInserting method at least once, and you must also use the weave method at least once (the results of these method cannot be discarded). You may also add more methods if you desire but you will need to turn in the modified files.
Extra Credit (+10pts)
Add in another instrument to your song for extra credit. To add another instrument, you will need to use multiple parts. Make sure the instruments are different and the riffs they are using are different (So if you use piano first then use drums, a guitar, etc... and make the riffs different)
Good References
Some possible song ideas
- Topgun Theme Song
- Great Balls of Fire
- Danger Zone
- Holiday Songs
- Mission Impossible
- Phil Collins
- Star Wars Imperial March
- Bach
- Linus and Lucy
What to Turn In
- AdvancedSongPhrase
- Your song class
- Any modified source code files
Where to Turn In
Ask Questions on HW4-Spring08 here.
Back to the top
DUE DATE: Friday March 7th 7pm with 5 hour grace period
Provided Files: Person.java, TreeNode.java, royalty.txt, familytree.txt
Ask questions at Questions on HW5-Spring08.
DUE DATE: Friday March 14th 7pm with 5 hour grace period
Provided Files: TurtleCommand.java, TurtleDrawing.java, commands2.txt, moreCommands.txt
Hopefully by this point, you have had already some experience with file I/O (file input/output) and drawing with turtles. Now you will be required to write a program that takes in a text file of commands and translate those commands into turtle movements on a world.
Analyzing the Text File Format
You may assume the format of the text file will always be the type of the command first and then the parameters that command takes in. The commands you will be expected to handle are:
Command type | Parameters | What it does |
world | width, height | Creates the world based on parameters |
forward | distance | Moves the turtle forward based on parameter |
turn | degree | Turns the turtle based on parameter |
moveTo | x coordinate, y coordinate | Moves the turtle to a specific coordinate |
setHeading | heading | Sets the turtle’s heading to a specific degree where 0 is North, 90 is East, 180 is South and 270 is West. |
TurtleDrawing
In the TurtleDrawing class, you will be required to write one new constructor and three new methods: extractCommands, drawCommands, outputToFile and toString.
public TurtleDrawing(String turtleCommands)
The constructor will take in the file path of the turtle commands text file. First initialize the commands LinkedList to be an empty LinkedList of TurtleCommand objects (meaning you must use ). Then call the extractCommands method passing in the turtleCommands.
public void extractCommands(String turtleCommandsTextFile)
In this method you will need to use a BufferedReader, Scanner or similar class to actually read the data from the text file. Go through the text file and extract each line at a time. Assume the first line of the text file will always be a world command. You can assume that each line will contain only one command. The first item in the line will be the command type and followed by its parameters. You can also assume that each line is correct meaning that each command has the exact number of parameters it needs (as outlined in the previous table).
For each line, create a new TurtleCommand placing the command type and its parameters in that TurtleCommand. Then add that TurtleCommand to the big LinkedList of TurtleCommands called commands. Do this until you have extracted all of the commands.
public String toString()
This is the method to return a String representation of the commands LinkedList. You should create a String representation so that it is in the same format of the turtle commands text file, because doing so will make the outputToFile method easier.
public void drawCommands()
This is the method where you draw all of the commands in your commands list. Loop through your entire commands list and check the type of each TurtleCommand. If the command type is "world" then you have to create the world based on the parameters in that TurtleCommand, add a new Turtle to that world and show the world. If the command type is "forward" then call the forward method on the turtle you created before. You will do something similar with the "moveTo", the "turn" and the "setHeading" command types. Remember to call the provided pause method after each command so you can see the progression of the commands.
Command type | What to do |
world | Create a new world based on parameters Create a new turtle and put it on that world Show the world |
forward | Call the forward method based on parameters on the previously defined turtle |
turn | Call the turn method based on parameters on the previously defined turtle |
moveTo | Call the moveTo method based on parameters on the previously defined turtle |
setHeading | Call the setHeading method based on parameters on the previously defined turtle | Remember to pause after |
public void outputToFile(String outputFile)
In this method, you will save all of the TurtleCommands from the commands LinkedList to the incoming file name. You simply need to use a FileWriter that takes in the outputFile and write all of the commands from the commands LinkedList. Remember to close the FileWriter after you are done writing all of the commands. One way you can tell if your output file is in the right format is to pass it into the extractCommands method.
public void addMoreCommandsFromFile(String moreCommands)
In this method you will extract even more commands from another file and add them to the commands LinkedList. Assume that this incoming file will not have a world command (Hint: Can you use the extractCommands method again?).
Main method
In your main method create a new TurtleDrawing and give it the path to the turtle commands text file. Then call the drawCommands method and print out the toString of the TurtleDrawing. Lastly you should call the outputToFile method passing in "output.txt" as the parameter. If this file does not exist already, it will be created for you by FileWriter.
What to Turn In
Where to Turn In
Ask questions at Questions on HW6-Spring08.
DUE DATE: Friday March 28th 7pm with 5 hour grace period
Provided Files: TurtleCommand.java, commands2.txt, moreCommands.txt, TurtleDrawing.java
Ask questions at Questions on HW6-Spring08.
SIGN UP DUE DATE: Friday March 28th 7pm with 5 hour grace period
Read the rules provided in the pdf and sign up here: Homework 6 Demo Sign Ups
Ask questions at Questions on HW6-Spring08.
DUE DATE: Wednesday April 9th 7pm with 5 hour grace period
Provided Files: Wolves.zip, Traffic.zip
Ask questions at Questions on HW7-Spring08.
See T-Square for details.
Ask questions at Questions on HW8-Spring08.
DUE DATE: Monday February 11, 7pm
Back to the top
Provided Files: TurtleDance.java
Using Sounds and Turtles create a musical dance where Turtles move in choreographed pattern to Sounds.
Turtle Dance Requirements
You must use at least six Turtles and have them move in a choreographed dance. A choreographed dance is a composed of a discernible pattern of movements. You cannot merely just randomize the Turtles’ movements. Also the Turtles must dance on an appropriate background; this means the Turtles must be on a Picture!
Musical Requirements
Your musical composition must contain at least five different Sounds. At least one of the Sounds needs to be one you found yourself and must be submitted to T-Square along with the rest of your homework.
Overall Requirements
The entire piece must be at least twelve seconds long. There must be some interweaving between the Turtle dance movements and Sounds. Simply put, you cannot you just move the Turtles a little and then play twelve seconds of Sounds.
Main Method
You must write a main method in TurtleDance to test your dance with Sounds. Though we are not requiring you to write any specific methods, you should write with a better coding style by breaking your dance in to different methods and using loops.
Creativity
A great deal of your points will be based on creativity. Impress your TAs! In case you are not exactly the creative type, here are a couple of dances to consider:1. Dance Off
A rival gang of Turtles has challenged your gang of Turtles to a dance off. Each gang of Turtles take turns doing a dance in unison. On each turn the current gang of Turtles must repeat what the other gang did previously as well as add on new moves. There must be at least 2 turns meaning that each gang must go at least 2 times.
2. Turtle Waltz
Several Turtle couples have decided to do the waltz. For each couple, the partners must face each other and move together with the music. You must have at least 3 Turtle couples and an appropriate background.
3. Turtle Concert
Intersperse music Sounds with spoken word Sounds to create a soundtrack for the concert. Choose one from the following dances:
a. Superstar and Backup Dancers - A Turtle superstar is performing his/her latest song. Have at least 5 backup dancers while the Turtle superstar is singing his/her song. Remember the backup dancers’ dance is usually more elaborate than the superstar’s.
b. Boy/Girl Band - Each member of the boy/girl band take turns stepping in front to do a part of the vocals (and perhaps his/her own dance) while the others dance in unison.
c. Superstar, Backup Dancers, and Audience – The concept is basically just like Superstar and Backup Dancers, but instead you must have some Turtles in the audience that will also occasionally move, but how and when they move is really up to you.
What to Turn In
- TurtleDance.java
- One new sound file and any additional sound files not found in MediaSources
- (optional) background pictures not in MediaSources
- (optional) any other modified java class.
How to Turn In
Ask questions at Questions on BonusHW1-Spring08.
DUE DATE: Friday March 7th 7pm
Provided Files: TurtleCommand.java, TurtleDrawing.java, commands2.txt, moreCommands.txt
Hopefully by this point, you have had already some experience with file I/O (file input/output) and drawing with turtles. Now you will be required to write a program that takes in a text file of commands and translate those commands into turtle movements on a world.
Analyzing the Text File Format
You may assume the format of the text file will always be the type of the command first and then the parameters that command takes in. The commands you will be expected to handle are:
Command type | Parameters | What it does |
world | width, height | Creates the world based on parameters |
forward | distance | Moves the turtle forward based on parameter |
turn | degree | Turns the turtle based on parameter |
moveTo | x coordinate, y coordinate | Moves the turtle to a specific coordinate |
TurtleDrawing
In the TurtleDrawing class, you will be required to write one new constructor and three new methods: extractCommands, drawCommands and toString.
public TurtleDrawing(String turtleCommands)
The constructor will take in the file path of the turtle commands text file. First initialize the commands LinkedList to be an empty LinkedList of TurtleCommand objects (meaning you must use ). Then call the extractCommands method passing in the turtleCommands.
public void extractCommands(String turtleCommandsTextFile)
In this method you will need to use a BufferedReader, Scanner or similar class to actually read the data from the text file. Go through the text file and extract each line at a time. Assume the first line of the text file will always be a world command. You can assume that each line will contain only one command. The first item in the line will be the command type and followed by its parameters. You can also assume that each line is correct meaning that each command has the exact number of parameters it needs (as outlined in the previous table).
For each line, create a new TurtleCommand placing the command type and its parameters in that TurtleCommand. Then add that TurtleCommand to the big LinkedList of TurtleCommands called commands. Do this until you have extracted all of the commands.
public String toString()
This is the method to return a String representation of the commands LinkedList. You should create a String representation so that it is in the same format of the turtle commands text file, because doing so will make the outputToFile method easier.
public void drawCommands()
This is the method where you draw all of the commands in your commands list. Loop through your entire commands list and check the type of each TurtleCommand. If the command type is "world" then you have to create the world based on the parameters in that TurtleCommand, add a new Turtle to that world and show the world. If the command type is "forward" then call the forward method on the turtle you created before. You will do something similar with the "moveTo", the "turn" and the "setHeading" command types. Remember to call the provided pause method after each command so you can see the progression of the commands.
Command type | What to do |
world | Create a new world based on parameters Create a new turtle and put it on that world Show the world |
forward | Call the forward method based on parameters on the previously defined turtle |
turn | Call the turn method based on parameters on the previously defined turtle |
moveTo | Call the moveTo method based on parameters on the previously defined turtle |
Remember to pause after
Main method
In your main method create a new TurtleDrawing and give it the path to the turtle commands text file. Then call the drawCommands method and print out the toString of the TurtleDrawing.
What to Turn In
Where to Turn In
Ask questions at Questions on BonusMiniHW1-Spring08.
DUE DATE: Friday March 21th 7pm with 5 hour grace period
Provided Files: BrokenSuperTurtle.java
DUE DATE: Friday March 28th 7pm with 5 hour grace period
Ask questions at Questions on BonusMiniHW3-Spring08.
DUE DATE: Friday April 11th 7pm with 5 hour grace period
Provided Files: AbstractContactList.java, ContactEntry.java, ContactNode.java
Ask questions at Questions on BonusMiniHW4-Spring08.
Link to this Page