The spacecraft thrusters were expected to receive commands in the metric system, using the newton as a unit of force. The software running on Earth performed calculations in units of pound force, an imperial measure. As a result, the Earth station asked for about five times as much thrust as they should have.
The problem arose partly because the software was not properly tested before launch. The total project cost was $327 million.
Managing Mars
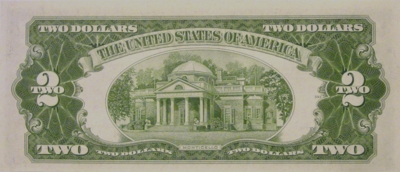
The first task, is, as always, to secure funding. Since the Monticello Gift Shop only traffics in two-dollar bills, we will need to know how many two-dollar bills to bring to ensure that we have enough to buy a $327 million JCO.
We'll start by defining a function that computes the answer for us. Type the following into DrScheme:
(define (how-many-twos dollars) (ceiling (/ dollars 2)))Check that it works by determining how many two dollar bills are required to have at least $10 and $7:
> (how-many-twos 10) 5 > (how-many-twos 7) 4The word define tells the interpreter that you are creating a new function. The word how-many-twos is the name we chose for the new function. The word dollars after the function name is the name we chose for its argument. The function body first divides the amount by two (/ dollars 2) and then uses the ceiling function to round up.
Your first task is to avoid the problem that befell the MCO by correctly converting pounds of force to newtons. For our purposes, 10 pounds of force are equal to 44.4822162 newtons.
Your next task is to correctly report on the idyllic climate of Monticello by converting from units of Fahrenheit to the more space-friendly units of Kelvin. Officially, the Kelvin value K is given by K = (F + 459.67) * (5/9), where the Fahrenheit value is F.
Testing
In this class you will be asked to write and turn in many programs. We will expect your programs to behave correctly. One of the best ways to achieve this is to understand what is required by each assignment, design and specify a program that would meet the requirements, and then use reason to develop a correct program. However, it is often nice to have a way to check your work.Testing an empirical approach for gaining confidence about the quality of a program. In essence, you test a program by running on some inputs for which you know the correct answer and comparing your programs outputs to those correct answers.
Ultimately, you are responsible for testing your work before you submit it. But we will provide you with some help ...
Automatic Adjudication
Once you believe you have defined your functions correctly and you have tested them a few times yourself, you can submit them to us for evaluation. The first step is to correctly attribute authorship on your assignment, as in:> (define author (list "wrw6y"))Use your official UVA ID instead of wrw6y. If you were working with a partner, you would also include your partner's email address:
> (define author (list "wrw6y" "mst3k"))
The second step is to save your definitions in a file. Use the File menu in DrScheme and choose Save Definitions or Save Definitions As. Once you have saved your Scheme definitions to a file, visit:
https://church.cs.virginia.edu/150/
If your browser gives you a security warning, go back and install the
US Higher Education Root Certificate
Note that you will need to log on using your university computing ID. Choose the relevant problem set -- currently you are working on Problem Set 0. Click on the Browse button, and select the Scheme definitions file you just saved. Then click on the Submit button. You should see output like this:
wrw6y PS0 Test Results
|
If you passed all of our tests, great! If not, take a look at the names of the tests you failed and try to infer what might have gone wrong. For example, in the results above (pound-force-to-newtons 0) worked fine but (pound-force-to-newtons 10) did not -- perhaps we are dividing when we should be multiplying? You may resubmit as often as you like before the deadline.
Make sure use the Check Syntax button in DrScheme before submitting. If you submit a program with a typo in it, the automatic adjudicator will notice, but will be fairly cryptic about it. For example, imagine you had this function definition:
; this is my comment (define (pound-force-to-newtons pf) (+ pf 4.44822162)))If you look closely, you'll note that there are too many closing parentheses on the third line. If we submit this file, we'll get the error message:
wrw6y PS0 Test Resultserror while processing your file#(struct:exn:fail:read "program:3:26: read: unexpected `)'" #did you check the syntax before submitting? |
The program:3:26 means "the problem may be on the third line of your program, and on the 26th character of that line". And in this example, it is. But you should always use DrScheme to check your Scheme syntax, since it is much friendlier.
Keep thinking about, fiddling with, testing and modifying your program until to submit it and get a score you are happy with. Consult with the TAs for help if desired (see the Collaboration Policy for this assignment, above). When you are finished, use the View my stored results link to check the results we have saved for you.
One of our goals with Automatic Adjudication is to make the grading as fair and transparent to you as possible. There should not be any surprises about your grade. Since you may resubmit as often as you like and the grading is direct, requests for official regrades will be met with a reasonable amount of skepticism.
Surveys and Administration
CS 150 traditionally attracts a diverse set of students with varied backgrounds -- not all of them are "pure CS". For example, of the first 71 people to sign up for the course in 2009, 31 had declared Psychology or Cognitive Science as a major while only 12 had declared Computer Science as a major.It is critically important that the course staff understand the needs and expectations of the students enrolled in the course. To that end, you are required to fill out a short Introductory Survey. You can find it on the Automatic Adjudication page. When you have turned it in, check that we have received it by requesting a summary.
In addition, we take Honor fairly seriously at Virginia. This course features a single honor pledge, rather than individual pledges for every assignment. Please sign it and turn it in as requested.
Teaser: Astronomy and Computer Science
The exploration of space touches on many computer science issues, some of which we will return to later in the course. Correctly programming spacecraft is still of critical importance: as reasonable as it might seem to merely "send all of the data back to earth", in practice bandwidth limitations and the speed of light delay prevent spacecraft from sending back everything and reacting to dynamic events. Machine Learning is a subfield of artificial intelligence concerned with extracting rules and patterns from massive datasets. For example, machine learning is used to help detect when your credit card has been stolen (based on anomalous purchases) and to recommend purchases on Amazon or Netflix (based on what you and others have enjoyed). Scientists from the NASA Jet Propulsion Laboratory have been experimenting with using machine learning on spacecraft, such as Earth Observing 1 and Mars Odyssey. In this area, machine learning helps scientists deal with uncalibrated data, noisy observations and limited spacecraft computing power. In 2007, Rebecca Castano et al. described how the use of machine learning on spacecraft confirmed the existence of a water ice annulus around the north polar cap of Mars.
Extra Credit: Rocketing to Mars
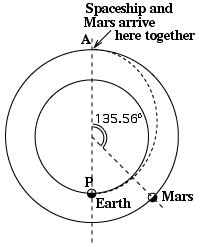
The solar system is dominated by the Sun's gravity, and in general objects moving in the solar system sweep out orbits that are parts of conic sections, as governed by Kepler's Laws. With that in mind, we might time the launch of our JCO rocket in such a way that when it reaches the orbital distance of Mars, the red planet is just moving in to the right position. This maneuver is called a Hohmann transfer orbit and was described by the German scientist Walter Hohmann in 1925. Your task is to write a function that will figure out how long such a trip will take to reach a given planet.
Kepler's Third Law states that for all objects orbiting the Sun, T2 / a3 = c, where T is the orbital period, a is the semi-major axis, and c is a constant. The semi-major axis is half the length of the orbital ellipse: if the orbit is circular, a is equal to the radius. The value of c is the same for all objects orbiting the Sun, including Earth and Mars. Its exact value depends on the units used. Since we're masters of unit conversions after Problem Set 0, we can simplify things dramatically by picking the right units. If we measure T in years and a in astronomical units, then for Earth T=1 and a=1, which forces c=1 as well. As a result, we can multiply both sides of the original equation by a3 and obtain T2 = a3. This holds for any orbit around the Sun, including the orbit of the rocket we'll use to fly the JCO to Mars.
In the diagram, the dotted line indicates the path our rocket will travel: starting from Earth at the bottom it sweeps out one half of an ellipse. The rocket and Mars arrive at point A at the same time. The length of the major axis of the rocket's ellipse is the distance from the Earth (at point P) to the Sun plus then the distance from the Sun to Mars. If the distance to Mars is r, then the major axis is 1 + r (recall that the distance from the Earth to the Sun is 1 when we measure in astronomical units). The length of the semimajor axis a is just half the length of the major axis: a = (1+r)/2. Since T2 = a3, you can now solve for T. The final complication is that T is the time to get there and back again (one complete elliptical orbit). We only want half of that time -- just to get from Earth to Mars.
Define a function hohmann-time that takes a single parameter r (the distance from the target planet to the Sun). It should return the number of years required for a one-way Hohmann transfer orbit from Earth to that planet, using the equations given above. Here are some hints:
> (* 10 10 10) 1000 > (sqrt 4) 2You may find it convenient to define two functions to solve this problem: a helper function that computes T given a, and hohmann-time that computes a and calls your helper function.
Extra Credit: Automatic Adjudication
Some problem sets will have an extra credit section at the end. The extra credit is, by definition, not required. The extra credit questions are targeted at students who want to explore the concepts a bit further and for students with a more previous experience in computing. The weight of an extra credit section is around 10% of the weight of its associated problem set.
cs150: Computer Science
from Ada and Euclid to Quantum Computing and the World Wide Web
Instructor
Westley Weimer
Teaching Assistants
Zak Fry
Paul DiOrio
Rachel Lathbury
Email Address
cs150-staff@cs.virginia.edu
Mondays and Wednesdays, 3:30-4:45pm in MEC 341
Wednesdays, 7:00-8:00pm and 8:00-9:00pm in OLS 001
(Small Hall Lab)
Monday 5:00-6:00 (Zak)
Tuesday 3:15-4:15 (Rachel)
Thursday 5:00-6:00 (Paul)
Sunday 3:00-4:00 (on request)
(Small Hall Lab)
Monday 2:00-3:00 (Rachel)
Tuesday 11:00-12:00 (Wes in Olsson 219)
Tuesday 3:00-4:00 (Zak)
Wednesday 1:00-2:00 (Paul)