Midterm Exam 2 Review-Fall 2007
Below are questions like those I plan to ask on Midterm REMOVED (Nov. 9). The exam will consist of 4 or 5 questions like these.
Please do try these questions! Post your answers, questions, comments, concerns, and criticisms on the pages for each question. Those comments, questions, etc. can also be about each others' answers! If someone posts an answer that you don't understand, ask about it! If you see a question here that you know the answer to, don't keep it to yourself – help your fellow students!
I will be reading your answers. Here are the ground rules for the interaction.
- If you don't post, neither will I. I will not be posting official solutions to these problems at all! If one of you gets it right, terrific!
- I will try to always point out if an answer is wrong. I won't always point out when the answer is right.
- I am glad to work with you toward the right answer. I will give hints, and I'm glad to respond to partial guesses.
Write insertAfterNext
Write the method insertAfterNext which takes an input node and inserts it after the current node’s next. If A’s getNext is B (A->B) and B’s getNext is D (A-B-D), and we call A.insertAfterNext(C), then the result should be A-B-C-D.
NOTE: Be sure to consider the possibility that this.getNext() is null! If this’s getNext is null, just insert the input after this. So, if A’s getNext is null, and we call A.insertAfterNext(C), we should get A-C.
You may found the the insertAfter code from the class LLNode:
/***Insert the input node after this node.*@param node element to insert after this.
**/
public void insertAfter(LLNode node){
// Save what "this" currently points at
LLNode oldNext = this.getNext();
this.setNext(node);
node.setNext(oldNext);
}
Questions, comments, answers at Exam 2 Review Fall2007:Write insertAfter
Understanding inheritance
Given the following code:
public class Superhero {
public String name;
public int strength;
public Superhero() {
name = “Batman”;
strength = 10;
}
public void introduce() {
System.out.print(“Hello. I am “ + name + “. And “);
if(strength < 15) {
System.out.println(“I am weak.”);
} else {
System.out.println(“I am strong.”);
}
}
public class NinjaTurtle extends Superhero {
public NinjaTurtle(int i) {
super();
String[] turtles = {“Leonardo”, “Donatello”, “Raphael”, “Michaelangelo”};
name = turtles[i];
strength = 25;
}
public void introduce() {
System.out.println(“I’m “ + name);
}
}
What will the output of the following statements be?
> Superhero batman = new Superhero();
> Superhero leo = new NinjaTurtle(0);
> leo.introduce();
> batman.introduce();
1_ When you create a method in a subclass that overrides a method from the superclass, how do you invoke the superclass method's behavior? What constraints are there for doing this in a constructor of a subclass?
2_ What is the point of an abstract class?
3_ What are the big requirements for compilation when talking about Java inheritance? What method will get called if the method is defined in both child and parent classes?
Questions, comments, answers at Exam 2 Review Fall2007:Understanding inheritance
Draw the Object Structures
Draw the object structures (e.g., boxes and arrows) for the data structures resulting from these methods.
(Note: You can use any notation we used in class to draw the data structure for B.)
A. From SoundListTest.java
public void setUp4(){
FileChooser.setMediaPath("/Users/guzdial/cs1316/MediaSources/");
Sound s = null; // For copying in sounds
s = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundElement(s);
s = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
SoundElement one = new SoundElement(s);
root.repeatNext(one,4);
s = new Sound(FileChooser.getMediaPath("corkpop-tenth.wav"));
SoundElement two = new SoundElement(s);
root.insertAfter(two);
s = new Sound(FileChooser.getMediaPath("clap-q.wav"));
SoundElement three = new SoundElement(s);
two.last().repeatNext(three,3);
root.playFromMeOn();
}
B. From SoundTreeExample.java
public void setUp3() {
FileChooser.setMediaPath("/Users/guzdial/cs1316/MediaSources/");
Sound clap = new Sound(FileChooser.getMediaPath("clap-q.wav"));
Sound chirp = new Sound(FileChooser.getMediaPath("chirp-2.wav"));
Sound rest = new Sound(FileChooser.getMediaPath("rest-1.wav"));
Sound snap = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
Sound clink = new Sound(FileChooser.getMediaPath("clink-tenth.wav"));
Sound clave = new Sound(FileChooser.getMediaPath("clave-twentieth.wav"));
Sound gong = new Sound(FileChooser.getMediaPath("gongb-2.wav"));
Sound guzdial = new Sound(FileChooser.getMediaPath("guzdial.wav"));
Sound is = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundBranch();
SoundNode sn;
SoundBranch branch1 = new SoundBranch();
sn = new SoundNode(guzdial.append(is).append(snap));
branch1.addChild(sn);
sn = new SoundNode(clink.append(snap).append(clave));
branch1.addChild(sn);
sn = new SoundNode(guzdial.append(is).append(is));
branch1.addChild(sn);
root.addChild(branch1);
scaledBranch = new ScaleBranch(2.0);
sn = new SoundNode(clink.append(clave).append(gong));
scaledBranch.addChild(sn);
sn = new SoundNode(rest.append(chirp).append(clap));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
SoundBranch branch2 = new SoundBranch();
sn = new SoundNode(clap.append(is).append(snap));
branch2.addChild(sn);
sn = new SoundNode(clink.append(snap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(chirp.append(is).append(is));
branch2.addChild(sn);
scaledBranch = new ScaleBranch(0.5);
sn = new SoundNode(guzdial.append(is).append(gong));
scaledBranch.addChild(sn);
scaledBranch.addChild(branch2);
root.addChild(scaledBranch);
SoundBranch branch3 = new SoundBranch();
sn = new SoundNode(clap.append(clap).append(clave));
branch3.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch3.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch3.addChild(sn);
root.addChild(branch3);
root.playFromMeOn();
}
Questions, comments, answers at Exam 2 Review Fall2007:Draw the Object Structures
GUIs are trees
Look at the following code:
import java.awt.*;
import javax.swing.*;
/** Simple GUI Tree Example
* This is a GUI Tree Example designed to give you an idea
* of what we want you to do on the exam. We realize that
* there is not much material on this in the lecture slides,
* but a GUI Tree is very similar to the work you have done
* with "Sound Trees" (i.e. SoundBranch, etc)
**/
public class SimpleGUITreeExample extends JPanel{
public SimpleGUITreeExample(){
/** Since our class extends JPanel, it itself (this) is a
* JPanel as well. So let's set the layout to be a
* BorderLayout
**/
this.setLayout(new BorderLayout());
/** Let's start creating the panel to add into this
*/
//panel1 is a JPanel with a BorderLayout
JPanel panel1 = new JPanel();
panel1.setLayout(new BorderLayout());
//Here we create button1, button2, button3 which are all JButtons
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JButton button3 = new JButton("Button 3");
//We add button1, button2, button3 to panel1
panel1.add(button1, BorderLayout.NORTH);
panel1.add(button2, BorderLayout.CENTER);
panel1.add(button3, BorderLayout.SOUTH);
//Add panel1 to this
this.add(panel1, BorderLayout.NORTH);
//panel2 is a JPanel with a FlowLayout
JPanel panel2 = new JPanel();
panel2.setLayout(new FlowLayout());
//We create button4 and button5 which are JButtons
JButton button4 = new JButton("Button 4");
JButton button5 = new JButton("Button 5");
//Add button4 and button5 to panel2
panel2.add (button4);
panel2.add (button5);
//Add panel2 to this
this.add(panel2, BorderLayout.SOUTH);
}
public static void main (String[] args){
JFrame frame = new JFrame ("Simple GUI Tree Example");
frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE);
SimpleGUITreeExample tree = new SimpleGUITreeExample();
frame.getContentPane().add(tree);
frame.pack();
frame.setVisible(true);
}
}
Here is the tree structure for SimpleGUITreeExample
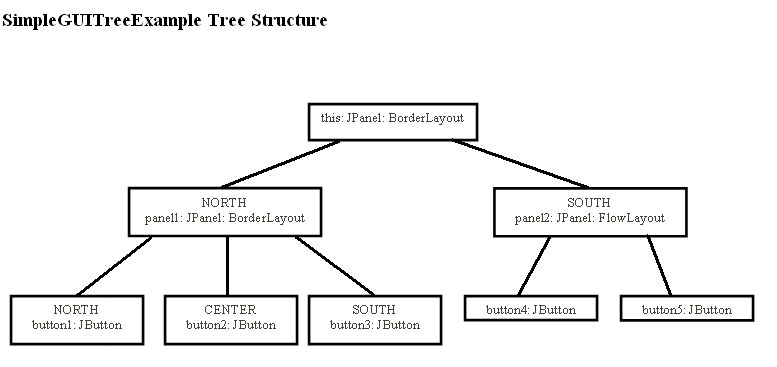
You are given the following class:
import java.awt.*;
import javax.swing.*;
public class ExampleGUI extends JPanel {
public ExampleGUI() {
JPanel buttonPanel = new JPanel();
JPanel contentPanel = new JPanel();
contentPanel.setLayout(new BorderLayout());
JPanel toSendTextPanel = new JPanel();
toSendTextPanel.setLayout(new BorderLayout());
JLabel receivedTextLabel = new JLabel("Received Messages");
JLabel toSendTextLabel = new JLabel("Text to Send");
JTextArea receivedText = new JTextArea("Jess: Hey Dawn, are you TAing next semester?\n"+
"Dawn: Nope. I'll be in sunny California getting a tan.\n"+
"Jess: Okay, but you have to get rid of that ugly farmer's tan.", 10, 10);
JTextArea toSendText = new JTextArea("Start typing here", 5, 10);
JButton warn = new JButton("Warn");
JButton chat = new JButton("Chat");
JButton send = new JButton("Send");
this.setLayout(new BorderLayout());
toSendTextPanel.add(toSendTextLabel, BorderLayout.NORTH);
toSendTextPanel.add(toSendText, BorderLayout.SOUTH);
buttonPanel.add(warn);
buttonPanel.add(chat);
buttonPanel.add(send);
contentPanel.add(receivedTextLabel, BorderLayout.NORTH);
contentPanel.add(receivedText, BorderLayout.CENTER);
contentPanel.add(toSendTextPanel, BorderLayout.SOUTH);
add(contentPanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
}
public static void main(String[] args) {
JFrame frame = new JFrame("IM");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ExampleGUI ex_gui = new ExampleGUI();
frame.getContentPane().add(ex_gui);
frame.pack();
frame.setVisible(true);
}
}
Draw the resulting GUI.
How would you represent the GUI structure as a tree?
Questions, comments, answers at Exam 2 Review Fall2007:GUIs are trees
More GUIs
1_ What are some different types of LayoutManagers. How do they handle the layout of their items?
2_ What is an ActionListener and what does it do?
3_ What are some other Listener interfaces? What do they do?
Questions, comments, answers at Exam 2 Review Fall2007:More GUIs
Java Keywords Faceoff
Give a short definition of each of the following words and say how they differ with their partner
extends v. implements
abstract v. concrete
static v. nonstatic (instance)
linked list v. array
stack v. queue
null v. void
super v. this
Questions, comments, answers at Exam 2 Review Fall2007:Java Keywords Faceoff
Links to this Page