Final Exam Review Fall2005
Below are questions like those we plan to ask on the Final Exam (Thursday 15 at 8:00 am). The exam will consist of 5-8 questions like these.
Please do try these questions! Post your answers, questions, comments, concerns, and criticisms on the pages for each question. Those comments, questions, etc. can also be about each others' answers! If someone posts an answer that you don't understand, ask about it! If you see a question here that you know the answer to, don't keep it to yourself – help your fellow students!
We will be reading your answers. Here are the ground rules for the interaction.
- If you don't post, neither will we. We will not be posting official solutions to these problems at all! If one of you gets it right, terrific!
- We will try to always point out if an answer is wrong. We won't always point out when the answer is right.
- We are glad to work with you toward the right answer. We will give hints, and I'm glad to respond to partial guesses.
Match the Data Structure
Below is a method setUp2() from SoundTreeExample.
(a) Below, you will find four possible diagrams describing the tree created in this method. Idnetify the one that you think is correct.
(b) Show that you understand how tree traversal works by describing the sounds that you hear, in order and with scaling effects, from playing this tree.
public void setUp2() {
FileChooser.setMediaPath("D:/cs1316/MediaSources/");
Sound clap = new Sound(FileChooser.getMediaPath("clap-q.wav"));
Sound chirp = new Sound(FileChooser.getMediaPath("chirp-2.wav"));
Sound rest = new Sound(FileChooser.getMediaPath("rest-1.wav"));
Sound snap = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
Sound clink = new Sound(FileChooser.getMediaPath("clink-tenth.wav"));
Sound clave = new Sound(FileChooser.getMediaPath("clave-twentieth.wav"));
Sound gong = new Sound(FileChooser.getMediaPath("gongb-2.wav"));
Sound guzdial = new Sound(FileChooser.getMediaPath("guzdial.wav"));
Sound is = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundBranch();
SoundNode sn;
SoundBranch branch1 = new SoundBranch();
sn = new SoundNode(guzdial.append(is).append(snap));
branch1.addChild(sn);
sn = new SoundNode(clink.append(snap).append(clave));
branch1.addChild(sn);
sn = new SoundNode(guzdial.append(is).append(is));
branch1.addChild(sn);
root.addChild(branch1);
scaledBranch = new ScaleBranch(2.0);
sn = new SoundNode(clink.append(clave).append(gong));
scaledBranch.addChild(sn);
sn = new SoundNode(rest.append(chirp).append(clap));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
scaledBranch = new ScaleBranch(0.5);
sn = new SoundNode(guzdial.append(is).append(gong));
scaledBranch.addChild(sn);
branch1.addChild(scaledBranch);
SoundBranch branch2 = new SoundBranch();
sn = new SoundNode(clap.append(clap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
root.addChild(branch2);
root.playFromMeOn();
}
Legend: 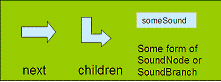
ONE: 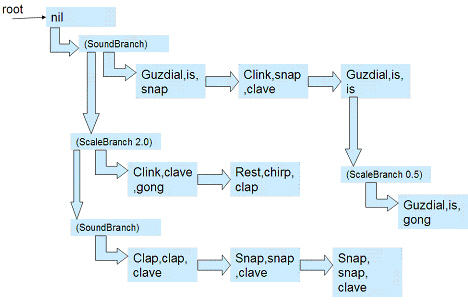
TWO: 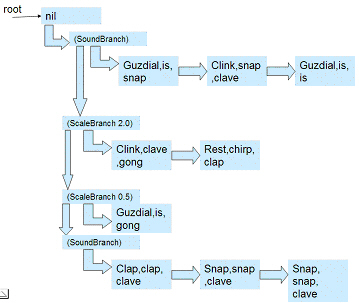
THREE: 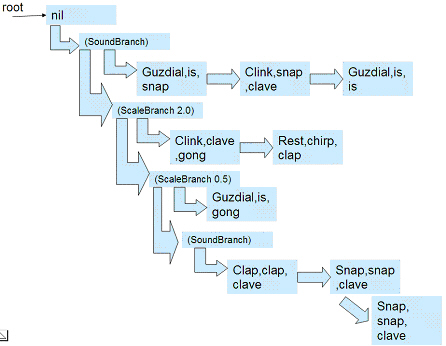
FOUR: 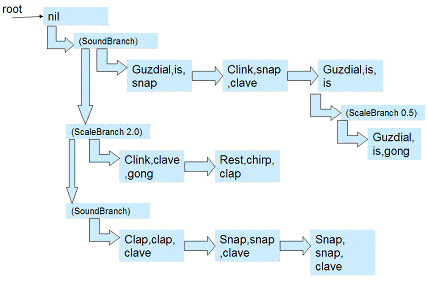
Questions, comments, and answers at FinalExam Review Fall2005: Match the Data Structure
Be a Data Structure Consultant
Now that you’re a data structures expert, people may want to hire you as a consultant to advise them on what data structure to use when. Suggest a structure for each of the situations described below. Recall that data structures that we discussed this semester include arrays, matrices, linked lists, circular linked lists, trees, graphs, stacks, queues, and event queues.
(a) “I’ve got a set of data that I need to store and access: Just a long list of objects. The size of the data will never change, and I need super-fast access to each and every element. What data structure should I use?”
(b) “I’m trying to represent the hierarchical relationship between employees in a company. People are being hired or leaving all the time. What’s a good way of representing that?”
(c) “I’m creating a simulation of a cafeteria. There are lines for sandwiches, for hot food, and for drinks. What’s the best data structure to represent the people standing in line?”
(d) “I’m going to represent the sidewalks around Georgia Tech. They go every which way, and they connect all over the place. What kind of data structure do you use to represent things like that?”
Questions, comments, and answers at FinalExam Review Fall2005: Be a Data Structure Consultant
What’s the Diff?
Each of the pairs below describes two things that are similar. Tell me what’s similar about them, and then, what the critical difference is it between them. For example, if I gave you int and double, you’d tell me that they are two types, each representing numbers, but int is only for integers and double can have decimal places.
(a) Discrete Event Simulations Continuous Simulations
(b) Stacks Queues
(c) Trees Lists
(d) BorderLayout FlowLayout
(e) Linked List Array
Questions, comments, and answers at FinalExam Review Fall2005: What’s the Diff?
Make a Super Truck
Below is the code for the Truck class from our FactorySimulation. Let’s imagine that our company has just bought a whole new fleet of super trucks that can carry 50% as much (20-30 items per load) and takes 1/3 less time to travel (average of two days per trip). What would you change in the Truck class to describe the new super trucks? Be specific—cross out and rewrite lines below so that this class will work as the new super truck.
import java.awt.Color; // For color
/**
* Truck -- delivers product from Factory
* to Warehouse.
**/
public class Truck extends DEAgent {
/////// Constants for Messages
public static final int FACTORY_ARRIVE = 0;
public static final int WAREHOUSE_ARRIVE = 1;
////// Fields /////
/**
* Amount of product being carried
**/
public int load;
//// METHODS ///
/** A new load is between 10 and 20 on a uniform distribution */
public int newLoad(){
return 10+randNumGen.nextInt(11);
}
/** A trip distance averages 3 days */
public double tripTime(){
double delay = randNumGen.nextGaussian()+3;
if (delay < 1)
// Must take at least one day
{return 1.0+((DESimulation) simulation).getTime();}
else {return delay+((DESimulation) simulation).getTime();}
}
/**
* Set up the truck
* Start out at the factory
**/
public void init(Simulation thisSim){
// Do the default init
super.init(thisSim);
this.setPenDown(false); // Pen up
this.setBodyColor(Color.green); // Let green deliver!
// Show the truck at the factory
this.moveTo(30,350);
// Load up at the factory, and set off for the warehouse
load = this.newLoad();
((DESimulation) thisSim).addEvent(
new SimEvent(this,tripTime(),WAREHOUSE_ARRIVE));
}
/**
* Process an event.
* Default is to do nothing with it.
**/
public void processEvent(int message){
switch(message){
case FACTORY_ARRIVE:
// Show the truck at the factory
((DESimulation) simulation).log(this.getName()+"\t Arrived at factory");
this.moveTo(30,350);
// Load up at the factory, and set off for the warehouse
load = this.newLoad();
((DESimulation) simulation).addEvent(
new SimEvent(this,tripTime(),WAREHOUSE_ARRIVE));
break;
case WAREHOUSE_ARRIVE:
// Show the truck at the warehouse
((DESimulation) simulation).log(this.getName()+"\t Arrived at warehouse with load \t"+load);
this.moveTo(50,50);
// Unload product -- takes zero time (unrealistic!)
((FactorySimulation) simulation).getFactory().add(load);
load = 0;
// Head back to factory
((DESimulation) simulation).addEvent(
new SimEvent(this,tripTime(),FACTORY_ARRIVE));
break;
}
}
// CONSTRUCTORS GO HERE, BUT THEY’VE BEEN REMOVED FOR THE EXAM.
}
Questions, comments, and answers at FinalExam Review Fall2005: Make a Super Truck
From Decrease to Gray
The below method decreases read for the target picture, as in myPic.decreaseRed().
/**
* Method to decrease the red by half in the current picture
*/
public void decreaseRed()
{
Pixel pixel = null; // the current pixel
int redValue; // the amount of red
// get the array of pixels for this picture object
Pixel[] pixels = this.getPixels();
// start the index at 0
int index = 0;
// loop while the index is less than the length of the pixels array
while (index < pixels.length)
{
// get the current pixel at this index
pixel = pixels[index];
// get the red value at the pixel
redValue = pixel.getRed();
// set the red value to half what it was
redValue = (int) (redValue * 0.5);
// set the red for this pixel to the new value
pixel.setRed(redValue);
// increment the index
index++;
}
}
Recall that a grayscale results from setting R, G, and B all to the same value, and that averaging red, green, and blue is a reasonable way of computing luminance.
Write grayscale() to change the picture to grayscale.
Questions, comments, and answers at Student215
Properties of Data Structures
Data structures that we discussed this semester include arrays, matrices, linked lists, circular linked lists, trees, graphs, stacks, queues, and event queues.
One or more data structures match each of the following statements or descriptions. List the data structures for which the statement is true:
A. Can change size dynamically to match the size of the data.
B. Is good for representing hierarchy.
C. Is particularly hard to insert into the middle of.
D. Can take a while to find an element in this data structure.
E. Is good for representing a line of people waiting for a ferris wheel ride.
F. Is good for representing animation states of a character.
G. Is used for representing the samples in a sound.
H. Is used for representing the pixels in a picture.
I. Might be used to represent the New York City Subway System
J. Is useful for reversing a list quickly
Questions, comments, and answers at FinalExam Review Fall2005: Properties of Data Structures
And a One and a Two
Below is the code from SongNode for weave. Write a new method weaveTwo which takes two input SongNode instances (one and two) and an int named count. For count times, insertAfter one copy of one and two copies of two.
/**
* Weave the input phrase count times every skipAmount nodes
* @param nextOne node to be copied into the list
* @param count how many times to copy
* @param skipAmount how many nodes to skip per weave
*/
public void weave(SongNode nextOne, int count, int skipAmount)
{
SongNode current = this; // Start from here
SongNode copy; // Where we keep the one to be weaved in
SongNode oldNext; // Need this to insert properly
int skipped; // Number skipped currently
for (int i=1; i <= count; i++)
{
copy = nextOne.copyNode(); // Make a copy
//Skip skipAmount nodes
skipped = 1;
while ((current.next() != null) && (skipped < skipAmount))
{
current = current.next();
skipped++;
};
if (current.next() == null) // Did we actually get to the end early?
break; // Leave the loop
oldNext = current.next(); // Save its next
current.insertAfter(copy); // Insert the copy after this one
current = oldNext; // Continue on with the rest
}
}
Questions, comments, and answers at FinalExam Review Fall2005: And a One and a Two
Draw the Tree
Below is a method from SoundTreeExample. Draw the data structure that it defines. BE SURE TO LABEL children versus next nodes!
public void setUp2() {
FileChooser.setMediaPath("D:/cs1316/MediaSources/");
Sound clap = new Sound(FileChooser.getMediaPath("clap-q.wav"));
Sound chirp = new Sound(FileChooser.getMediaPath("chirp-2.wav"));
Sound rest = new Sound(FileChooser.getMediaPath("rest-1.wav"));
Sound snap = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
Sound clink = new Sound(FileChooser.getMediaPath("clink-tenth.wav"));
Sound clave = new Sound(FileChooser.getMediaPath("clave-twentieth.wav"));
Sound gong = new Sound(FileChooser.getMediaPath("gongb-2.wav"));
Sound guzdial = new Sound(FileChooser.getMediaPath("guzdial.wav"));
Sound is = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundBranch();
SoundNode sn;
SoundBranch branch1 = new SoundBranch();
sn = new SoundNode(guzdial.append(is).append(snap));
branch1.addChild(sn);
sn = new SoundNode(clink.append(snap).append(clave));
branch1.addChild(sn);
sn = new SoundNode(guzdial.append(is).append(is));
branch1.addChild(sn);
root.addChild(branch1);
scaledBranch = new ScaleBranch(2.0);
sn = new SoundNode(clink.append(clave).append(gong));
scaledBranch.addChild(sn);
sn = new SoundNode(rest.append(chirp).append(clap));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
scaledBranch = new ScaleBranch(0.5);
sn = new SoundNode(guzdial.append(is).append(gong));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
SoundBranch branch2 = new SoundBranch();
sn = new SoundNode(clap.append(clap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
sn = new SoundNode(snap.append(snap).append(clave));
branch2.addChild(sn);
root.addChild(branch2);
root.playFromMeOn();
}
Questions, comments, and answers at FinalExam Review Fall2005: Draw the Tree
Draw a Wolf Tree
Below is the set-up code from WolfAttackMovie. Draw the data structure that it defines.
public void setUp(){
FileChooser.setMediaPath("D:/cs1316/mediasources/");
Picture wolf = new Picture(FileChooser.getMediaPath("dog-blue.jpg"));
Picture house = new Picture(FileChooser.getMediaPath("house-blue.jpg"));
Picture tree = new Picture(FileChooser.getMediaPath("tree-blue.jpg"));
Picture monster = new Picture(FileChooser.getMediaPath("monster-face3.jpg"));
//Make the forest
MoveBranch forest = new MoveBranch(10,400); // forest on the bottom
HBranch trees = new HBranch(50); // Spaced out 50 pixels between
BlueScreenNode treenode;
for (int i=0; i < 8; i++) // insert 8 trees
{treenode = new BlueScreenNode(tree.scale(0.5));
trees.addChild(treenode);}
forest.addChild(trees);
// Make the cluster of attacking "wolves"
wolfentry = new MoveBranch(10,50); // starting position
VBranch wolves = new VBranch(20); // space out by 20 pixels between
BlueScreenNode wolf1 = new BlueScreenNode(wolf.scale(0.5));
BlueScreenNode wolf2 = new BlueScreenNode(wolf.scale(0.5));
BlueScreenNode wolf3 = new BlueScreenNode(wolf.scale(0.5));
wolves.addChild(wolf1);wolves.addChild(wolf2);wolves.addChild(wolf3);
wolfentry.addChild(wolves);
// Make the cluster of retreating "wolves"
wolfretreat = new MoveBranch(400,50); // starting position
wolves = new VBranch(20); // space them out by 20 pixels between
wolf1 = new BlueScreenNode(wolf.scale(0.5).flip());
wolf2 = new BlueScreenNode(wolf.scale(0.5).flip());
wolf3 = new BlueScreenNode(wolf.scale(0.5).flip());
wolves.addChild(wolf1);wolves.addChild(wolf2);wolves.addChild(wolf3);
wolfretreat.addChild(wolves);
// Make the village
MoveBranch village = new MoveBranch(300,450); // Village on bottom
HBranch hhouses = new HBranch(40); // Houses are 40 pixels apart across
BlueScreenNode house1 = new BlueScreenNode(house.scale(0.25));
BlueScreenNode house2 = new BlueScreenNode(house.scale(0.25));
BlueScreenNode house3 = new BlueScreenNode(house.scale(0.25));
VBranch vhouses = new VBranch(-50); // Houses move UP, 50 pixels apart
BlueScreenNode house4 = new BlueScreenNode(house.scale(0.25));
BlueScreenNode house5 = new BlueScreenNode(house.scale(0.25));
BlueScreenNode house6 = new BlueScreenNode(house.scale(0.25));
vhouses.addChild(house4); vhouses.addChild(house5); vhouses.addChild(house6);
hhouses.addChild(house1); hhouses.addChild(house2); hhouses.addChild(house3);
hhouses.addChild(vhouses); // Yes, a VBranch can be a child of an HBranch!
village.addChild(hhouses);
// Make the monster
hero = new MoveBranch(400,300);
BlueScreenNode heronode = new BlueScreenNode(monster.scale(0.75).flip());
hero.addChild(heronode);
//Assemble the base scene
sceneRoot = new Branch();
sceneRoot.addChild(forest);
sceneRoot.addChild(village);
sceneRoot.addChild(wolfentry);
}
Questions, comments, and answers at FinalExam Review Fall2005: Draw a Wolf Tree
Compare the GUIs
A. Draw the tree described by the below two classes.
B. Explain the difference in what the user sees from creating instances of these two classes. Relate it to trees and rendering.
EXAMPLE ONE:
/**
* A GUI that has various components in it, to demonstrate
* UI components and layout managers (rendering)
**/
import javax.swing.*; // Need this to reach Swing components
import java.awt.*; // Need this to reach FlowLayout
public class GUItreeFlowed extends JFrame {
public GUItreeFlowed(){
super("GUI Tree Flowed Example");
this.getContentPane().setLayout(new FlowLayout());
/* Put in a panel with a label in it */
JPanel panel1 = new JPanel();
this.getContentPane().add(panel1);
JLabel label = new JLabel("This is panel 1!");
panel1.add(label);
/* Put in another panel with two buttons in it */
JPanel panel2 = new JPanel();
this.getContentPane().add(panel2);
JButton button1 = new JButton("Make a sound");
panel2.add(button1);
JButton button2 = new JButton("Make a picture");
panel2.add(button2);
this.pack();
this.setVisible(true);
}
EXAMPLE TWO:
/**
* A GUI that has various components in it, to demonstrate
* UI components and layout managers (rendering)
**/
import javax.swing.*; // Need this to reach Swing components
import java.awt.*; // Need this to reach BorderLayout
public class GUItreeBordered extends JFrame {
public GUItreeBordered(){
super("GUI Tree Bordered Example");
this.getContentPane().setLayout(new BorderLayout());
/* Put in a panel with a label in it */
JPanel panel1 = new JPanel();
this.getContentPane().add(panel1,BorderLayout.NORTH);
JLabel label = new JLabel("This is panel 1!");
panel1.add(label);
/* Put in another panel with two buttons in it */
JPanel panel2 = new JPanel();
this.getContentPane().add(panel2,BorderLayout.SOUTH);
JButton button1 = new JButton("Make a sound");
panel2.add(button1);
JButton button2 = new JButton("Make a picture");
panel2.add(button2);
this.pack();
this.setVisible(true);
}
}
Questions, comments, and answers at FinalExam Review Fall2005: Compare the GUIs
Slow! Sick People Crossing!
Below is the code for the class PersonAgent from the DiseaseSimulation example. REMOVEDge it so that, when people get sick, they slow down by 75%.
import java.awt.Color; // Color for colorizing
import java.util.LinkedList;
/**
* PersonAgent -- Person as a subclass of Agent
**/
public class PersonAgent extends Agent {
public boolean infection;
/**
* Initialize, by setting color and making move fast
**/
public void init(Simulation thisSim){
// Do the normal initializations
super.init(thisSim);
// Make it lightGray
setColor(Color.lightGray);
// Don't need to see the trail
setPenDown(false);
// Start out uninfected
infection = false;
// Make the speed large
speed = 100;
}
/**
* Count infected
**/
public int infected() {
int count = 0;
LinkedList agents = simulation.getAgents();
PersonAgent check;
for (int i = 0; i<agents.size(); i++){
check = (PersonAgent) agents.get(i);
if (check.infection) {count++;}
}
return count;
}
/**
* Become infected
**/
public void infect(){
this.infection = true;
this.setColor(Color.red);
// Print out count of number infected
System.out.println("Number infected: "+infected());
}
/**
* How a Person acts
**/
public void act()
{
// Is there a person within infection range of me?
PersonAgent closePerson = (PersonAgent) getClosest(20,
simulation.getAgents());
if (closePerson != null) {
// If this person is infected, and I'm not infected
if (closePerson.infection && !this.infection) {
// I become infected
this.infect();
}
}
// Run the normal act() -- wander aimlessly
super.act();
}
////////////////////////////// Constructors ////////////////////////
// Copy this section AS-IS into subclasses, but rename Agent to
// Your class.
/**
* Constructor that takes the model display (the original
* position will be randomly assigned)
* @param modelDisplayer thing that displays the model
* @param thisSim my simulation
*/
public PersonAgent (ModelDisplay modelDisplayer,Simulation thisSim)
{
super(randNumGen.nextInt(modelDisplayer.getWidth()),
randNumGen.nextInt(modelDisplayer.getHeight()),
modelDisplayer, thisSim);
}
/** Constructor that takes the x and y and a model
* display to draw it on
* @param x the starting x position
* @param y the starting y position
* @param modelDisplayer the thing that displays the model
* @param thisSim my simulation
*/
public PersonAgent (int x, int y, ModelDisplay modelDisplayer,
Simulation thisSim)
{
// let the parent constructor handle it
super(x,y,modelDisplayer,thisSim);
}
}
Questions, comments, and answers at FinalExam Review Fall2005: Slow! Sick People Crossing!
Explain It in Your Words
A. What's the difference between discrete event and continuous simulations? When would you use each? For each, give an example of a kind of question that you could ask with one but not with the other.
B. Why did we use trees to create animations?
C. Why do we consider it "hard" to insert and delete into the middle of an array? Is it easier or harder with a matrix?
Questions, comments, and answers at FinalExam Review Fall2005: Explain It in Your Words
Interpret the UML
Below is the UML class diagram for the Factory discrete event simulation.
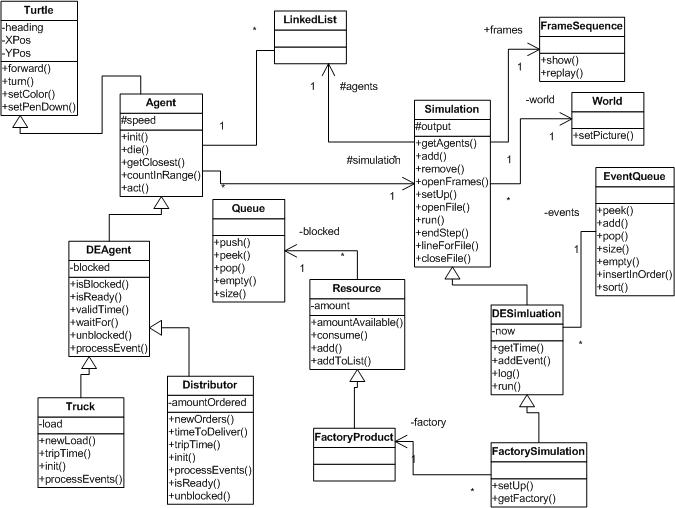
A. By what name does a Resource know its queue of agents waiting for the resource?
B. When an instance of Distributor wants to get the object representing the factory, what expression does it evaluate? (Yes, it all is here in the diagram.)
C. If a Truck wanted to check the position of all trucks and distributors, how would it get at all of those objects? What expression would it evaluate?
D. By what name does FactorySimulation access an instance of FrameSequence?
Questions, comments, and answers at FinalExam Review Fall2005: Interpret the UML
Links to this Page