Practice Exam 1-Summer07
A. Match the following terms with their descriptions:
1. double
2. int
3. char
4. boolean
5. String
6. void
7. null
8. final
|
a. Used to describe a method that does not return anything
b. A data type that can only hold true or false values
c. Equivalent to an empty object, or nothing
d. A data type that can hold multiple characters
e. Describes something that cannot be altered after declaration
f. A data type that can only hold integer values
g. A data type that can hold decimal values
h. A data type that can only hold single characters such as a, b and c
|
Ask questions at Practice Exam 1-Summer07:Matching
B. Select the correct answer:
Use the following code to answer questions 1 and 2. Turtle[] turtleArray = new Turtle[10];
1.How many Turtles can turtleArray hold?
a. 8
b. 9
c. 10
d. 11
e. Not enough information is given
2. How many Turtles exist in turtleArray right now?
a. 0
b. 1
c. 10
d. 11
e. Not enough information is given
3. Sarah wants to create a new subclass of the Nissan class called Nissan350Z. Which of the following is the correct class header for the Nissan350Z class?
a. public class Nissan350Z{
}
b. public class Nissan350Z implements Nissan{...}
c. public class Nissan350Z extends Nissan{
}
d. public class Nissan extends Nissan350Z{
)
e. public class Nissan implements Nissan350Z{
}
4. Which of the following would be considered a constructor of the class Elephant?
a. public void makeAnElephant() {
}
b. public Elephant(int age) {
}
c. public elephant(){
}
d. public void Elephant(){
}
e. public makeAnElephant(){
}
5. Consider the following line of code: World universe = new World();
Turtle leonardo = new Turtle(universe);
Why are World and Turtle capitalized while universe and leonardo not?
a. Classes in Java must be capitalized while variables cannot be. If you do not follow this, the Java compiler will throw errors.
b. Variables in Java must be capitalized while classes cannot be. If you do not follow this, the Java compiler will throw errors.
c. We only capitalize the type of the variable and not the variable itself.
d. It is just a convention (a rule that is socially enforced) that everyone follows. Actually it does not matter which one is capitalized or not.
e. Variables in Java must be capitalized while classes cannot be. If you do not follow this, the Java compiler will throw exceptions.
6. What does Math.random() return?
a. (-1,1) random doubles value -1 and 1, but excluding -1 and 1
b. (0,1] random double value between 0 and 1 including 1, but excluding 0
c. (0,1) random double value between 0 and 1 excluding 0 and 1
d. [0,1) random double value between 0 and 1 including 0, but excluding 1
e. [0,1] random double value between 0 and 1 including 0 and 1
Ask question at Student703
Use the following code to help you with part C: public void reduceBlue(){
Pixel[] pixels = this.getPixels();
Pixel pixel = null;
int value = 0;
for (int i = 0; i < pixels.length; i++) {
pixel = pixels[i];
value = pixel.getBlue();
pixel.setBlue((int) (value *0.7));
}
}
C. Write a Picture method called negateEveryOtherPixel() that negates every other pixel in the Picture. Remember that in order to negate the pixel, you set the pixel to 255 minus the original value. You must use a while loop in your solution.
public void negateEveryOtherPixel(){
}
Ask question at Practice Exam 1-Summer07:Picture Method
Use the following code to help you with part D: public class TurtleSquares {
public static void main(String [] args){
World world = new World();
Turtle t = new Turtle(world);
for (int i = 0; i 4; i++){
t.forward(100);
t.turn(90);
}
}
}
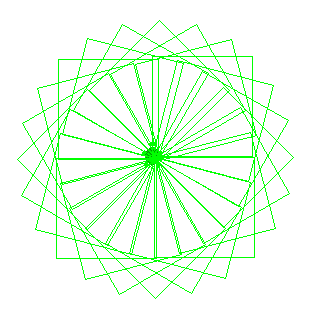
D. Within a class called TurtleBoxes write a main method to generate the picture shown above. You only need one Turtle to do that drawing. The Turtle turns 15 degrees each time before drawing a 100 by 100 pixel box. Think about how many times the Turtle must turn in order to cover all 360 degrees of a circle. You must use a loop (for or while)in your method. (See resources page for helpful code).
public class TurtleBoxes{
}
Ask question at Practice Exam 1-Summer07:Turtle Boxes
Using the following code to help you with part E: public String tossCoin() {
int value = (int)(2*Math.random());
if (value == 1)
return "Heads";
else
return "Tails";
}
E. Write a method called diceRollx3() that simulates the throw of 3 6-sided dice where the possible values are from 3 to 18. Think more what happens when you cast a double to an int and not really about partitioning. (See resources page for helpful code).
public int diceRollx3(){
}
Ask question at Practice Exam 1-Summer07:DiceRollx3
Links to this Page