Pre-Quiz3 - Fall 2006
Q1. Who's your grading TA? (Please circle your answer)
Dawn Joel Mark Brian REMOVEDng-Yen Richard
Q2. Here're the Student.java class and the Person.java class:
public class Student extends Person {
private String school;
private int schoolID;
public Student(String someName){
super(someName); // Must be the first line
this.defaultSchool();
}
public Student(String someName, int someID){
super(someName);
this.defaultSchool();
this.schoolID = someID;
}
public String getSchool(){
return this.school;
}
public void setSchool(String someSchool,int someID){
this.school = someSchool;
this.schoolID = someID;
}
public void defaultSchool(){
this.school = "Georgia Tech";
}
public String toString(){
return super.toString()+". And I'm a student at "
+this.getSchool()+" and my school ID is "+this.schoolID;
}
public void greet(){
super.greet();
System.out.println("Go away -- I'm late for class! I'm a student at " +this.getSchool());
}
}
public class Person {
private String name;
public Person(){
this.setName("Not-yet-named");}
public Person(String name){
this.setName(name);}
public String toString(){
return "Person named "+this.name;}
public void setName(String somename)
{this.name = somename;}
public String getName()
{return this.name;}
public void greet(){
System.out.println("Howdy! My name is " +this.name);}
}
If you were to execute the following main method, what would you see as the output?
public class Prequiz3 {
public static void main(String[] args){
//Create two instances of Student Object
Person mike = new Student("Mike");
Student fred = new Student("Fred");
((Student)mike).setSchool("Georgia Tech",10);
fred.setSchool("UGA",11);
System.out.println("Fred is " + fred);
mike.greet();
}
}
Q3. Consider the follwing SoundTreeExample class:
public class SoundTreeExample {
ScaleBranch scaledBranch; // Needed between methods
SoundBranch root;
public void setUp() {
FileChooser.setMediaPath("D:/cs1316/MediaSources/");
Sound clap = new Sound(FileChooser.getMediaPath("clap-q.wav"));
Sound chirp = new Sound(FileChooser.getMediaPath("chirp-2.wav"));
Sound rest = new Sound(FileChooser.getMediaPath("rest-1.wav"));
Sound snap = new Sound(FileChooser.getMediaPath("snap-tenth.wav"));
Sound clink = new Sound(FileChooser.getMediaPath("clink-tenth.wav"));
Sound clave = new Sound(FileChooser.getMediaPath("clave-twentieth.wav"));
Sound gong = new Sound(FileChooser.getMediaPath("gongb-2.wav"));
Sound guzdial = new Sound(FileChooser.getMediaPath("guzdial.wav"));
Sound is = new Sound(FileChooser.getMediaPath("is.wav"));
root = new SoundBranch();
SoundNode sn;
SoundBranch branch1 = new SoundBranch();
sn = new SoundNode(guzdial);
branch1.addChild(sn);
sn = new SoundNode(clink.append(snap));
branch1.addChild(sn);
root.addChild(branch1);
scaledBranch = new ScaleBranch(2.0);
sn = new SoundNode(clink);
scaledBranch.addChild(sn);
sn = new SoundNode(rest.append(chirp));
scaledBranch.addChild(sn);
root.addChild(scaledBranch);
SoundBranch branch2 = new SoundBranch();
sn = new SoundNode(clap);
branch2.addChild(sn);
sn = new SoundNode(snap.append(clave));
branch2.addChild(sn);
root.addChild(branch2);
root.playFromMeOn();
}
//Ignore the rest.
}
We'll execute this method like this:
> SoundTreeExample ste = new SoundTreeExample();
> ste.setUp();
Draw a diagram of what the nodes (branches, nodes, everything) connected to root will look like.
Be sure to label each node w/variable name, Class name, and sound.
(Note: Please use the following notation to distinguish the child and next references.)
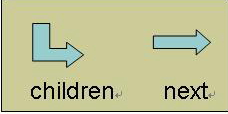
Comments, questions, and answers!
Link to this Page
- Pre-Quizzes-Fall 2006 last edited on 27 December 2006 at 5:08 pm by 24-241-99-170.static.stls.mo.charter.com