Also you can put your code in <code></code> tags to keep the formatting (so comments don't messup on the coweb) - Joel
but on the second part of your code, you start it from half way thru, dont we have to start it from the pixel (1,1)?
Chris A
(0,0) is the starting position. If you start it from (0,0) then it will write over the code where it copies the top half of the picture. I ran the code on a picture to make sure it works, but I'm not sure how to post a picture on coweb otherwise I'd post it so I could show better what this looks like.
REMOVED
Okay this code right here takes the original image, makes the target picture double the height of the original, and copies the original picture onto the top half of the target image. It then starts at that point and copies the mirror image to the bottom half of the target image (note the srcy = getHeight()-1 because if it starts at the height then technically it's already out of bounds).
Run it through MyPicture.java. just change swan.flip() to swan.horizontalMirror() and comment all the rest of the mumbo jumbo that's added to the end of it.
REMOVED Cormack
/**
* Method to mirror an image along the horizontal axis
**/
public Picture horizontalMirror()
{
Pixel currPixel;
Picture target = new Picture(this.getWidth(),this.getHeight()*2);
//This Loop copies directly the top half onto the tartet picture
for (int srcx = 0, trgx = 0; srcx < getWidth(); srcx++, trgx++)
{
for (int srcy = 0, trgy = 0; srcy < getHeight(); srcy++, trgy++)
{
// get the current pixel
currPixel = this.getPixel(srcx,srcy);
/* copy the color of currPixel into target
*/
target.getPixel(trgx,trgy).setColor(currPixel.getColor());
}
};
//This Loop creates the bottom half horizontally mirrored to the top half
for (int srcx = 0, trgx = 0; srcx < getWidth(); srcx++, trgx++)
{
for (int srcy = getHeight()-1, trgy = getHeight(); srcy > 0; srcy--, trgy++)
{
// get the current pixel
currPixel = this.getPixel(srcx,srcy);
/* copy the color of currPixel into target
*/
target.getPixel(trgx,trgy).setColor(currPixel.getColor());
}
};
return target;
}
hey, that is not what i thought the question ask. Anyway, this is what my interpretation of the question is:

Sorry for my bad code just now. I never compile it, so there is a lot of mistakes and regarding to the punctuation, i use C++ a lot, so forgive me! ;) here is my fixed code, which really works
public Picture test1()
{
Pixel abc;
//the reason i put +2 in the height part is to compensate the offset, if i put +1 or nothing, it wont work.
Picture target = new Picture(this.getWidth(), this.getHeight()+2);
for (int y = 0; y < this.getHeight()/2 + 1; y++)
{
for (int x=0; x < this.getWidth(); x++)
{
abc = this.getPixel(x,y);
target.getPixel(x,y).setColor(abc.getColor());
target.getPixel(x,(this.getHeight() + 1 - y)).setColor(abc.getColor());
}
}
return target;
}
What i really do is to copy one pixel and put it in two point, maybe you can also implement it in your code(doesnt matter if you are flipping it down), so u only need two loops instead of four.
//Chris Ang
I see where you're coming from now. I guess what we're not deciding on now is aesthetics and general coding preference. Looks to me like both of ours worked :).
Good luck on the test today.
REMOVED
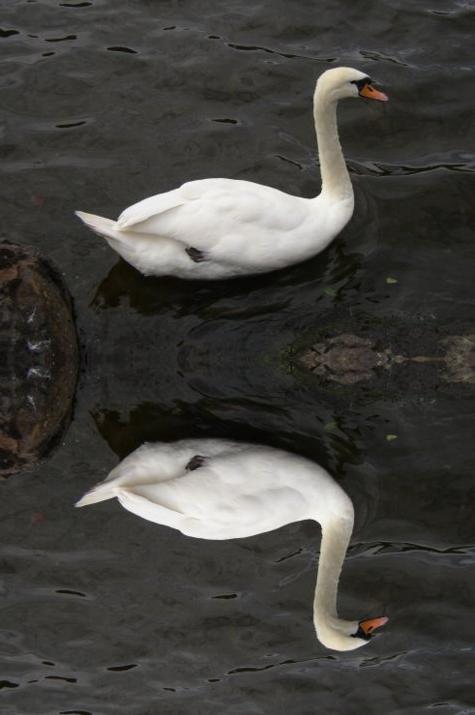
Link to this Page