Homework for Spring 2013
Homework #1: Manipulate Pictures
Due Jan 29
Write two new methods in the class Picture that implement some kind of image manipulation.The two methods can not be the same.
- You must use a for loop in one method and a while loop in the other!
- You must use random() at least once, in other words, calling the method twice on the same input should return different results.
- For one method, the picture that gets manipulated should be the target that receives the method (i.e., this). For example, if you implement the method "mytweak()", then you'll use it on some picture somePicture.mytweak(), and you'll expect the picture somePicture to change. (Don't bother returning a DIFFERENT picture for this method. Your method should be a void method.)
- For the second method, return a new changed picture. Your method should be a Picture method, and you must return the new method.
What manipulation that gets implemented is entirely up to you: Increasing blue while decreasing red, flipping vertically, posterizing, etc. You must implement something NOT already implemented in the class Picture. You can use the methods that are there as examples, but do not make any calls to those existing methods to implement your new methods.
Turn in Picture.java file via T-Square. Name your methods something that makes sense for what it does and place those names in the top comments region of the Picture.java class definition. Place your methods at the bottom of the class definition, with a comment before it indicating what your method does.
Priscilla will compile your Picture.java file in DrJava and test it using the interactions pane. She will create an instance of a Picture, execute each of your defined methods on that Picture object and show() the results.
WHAT TO TURN IN
- Modified Picture.java.
- Please include your name in the beginning comments
- Picture.java should contain 2 new methods, one method using a for loop, the other using a while loop with comments explaining what each does.
Grading Criteria
- Compiles with no errors: 45 points
- Use random(): 5 points
- Return void from one, changing this: 10 points
- Return Picture from the other: 10 points
- Has a working Picture method using FOR loop: 10 points
- Has a working Picture method using WHILE loop: 10 points
- Good comments on each: 10 points
Homework 2
Due Feb 6, 2013
Modify Picture.java so that when run (i.e., running the main() method), a collage is generated and shown (via .show). Here are the specs that you have to meet:
- You must create a canvas picture of 1024 x 768. All of your pictures must be composed into the canvas.
- The same picture must appear at least four times: Once in its original form, then three more times modified in some way.
- You must use scale at least twice.
- You must use one other manipulation method in Picture (e.g., negate or oilPaint or flip).
- You must use a turtle do drop() a picture at least once.
- You must create a new Picture manipulation method, and use that in your collage as well.
Submit Picture.java as your turn-in. You must use good commenting style. You are bound by the honor code not to share code with anyone.
Grading Rubric
- 40 points: Compiles (10), runs (from main method) (20), and generates a picture of 1024x768 (10).
- 10 points: Same picture appears 4 times.
- 10 points: Scale is used twice.
- 10 points: Use at least one other Picture method.
- 10 points: Uses a turtle with drop()
- 20 points: Create and use a new picture method.
Homework 3: Picture in a Picture
Due Feb 15 2013
Write a method for class Picture that takes a picture as input and places it inside the target picture. Call the method composeInAPicture. These are the inputs to the method:
- insertPicture: an instance of Picture to be inserted into the target picture.
- location: a String that is either "UL" for upper left, "LL" for lower left, "UR" for upper right, and "LR" for lower right.
- If the string is "UL," insert the picture at 0,0
- If the string is "LR," insert the picture so that it's lower right corner is at the maximum height and width of the target.
- If the string is "UR," insert the picture so that it's upper right corner is at x=width of the target and y=0.
- If the string is "LL," insert the picture so that it's lower left corner is at x=0 and y = height of the target
Scale the input picture so that it only takes up a maximum of 25% of the area of the original picture.
Here's an example of how you might use this:
> Picture canvas = new Picture(FileChooser.getMediaPath("barbara.jpg"))
> Picture swan = new Picture(FileChooser.getMediaPath("swan.jpg"))
> swan
Picture, filename /Users/guzdial/Desktop/MediaComp/mediasources/swan.jpg height 360 width 480
> canvas
Picture, filename /Users/guzdial/Desktop/MediaComp/mediasources/barbara.jpg height 294 width 222
> canvas.composeInAPicture(swan,"LR")
Here's what's going on inside the method:
// What is 25% of the height and width of the canvas?
> 0.25 294
73.5
>0.25 222
55.5
// What's the scaling for the 360 x 480 swan?
> 73.5 / 360
0.20416666666666666
> 55.5 / 480
0.115625
// Make a small enough swan, taking the minimum of those two numbers.
> Picture lilswan = swan.scale(0.11)
> lilswan
Picture, filename None height 40 width 53
We now want to compose the swan for "LR" so that it's at 222 (width of canvas) - 53 (width of lilswan), and 294 (height of canvas) - 40 (height of lilswan), which is essentially this:
> lilswan.compose(canvas,222-53,294-40)
With a result of:
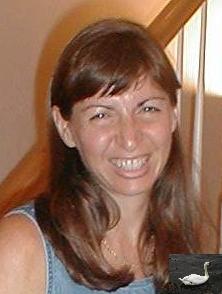
Submit Picture.java as your turn-in. You must use good commenting style. You are bound by the honor code not to share code with anyone.