Homework for Spring 2012
Homework #1: Manipulate Pictures
Write two new methods in the class Picture that implement some kind of image manipulation.The two methods can not be the same. You must use a for loop in one method and a while loop in the other! The picture that gets manipulated should be the target that receives the method (i.e., this). For example, if you implement the method "mytweak()", then you'll use it on some picture somePicture.mytweak(), and you'll expect the picture somePicture to change. (Don't bother returning a DIFFERENT picture for this method. In other words, we expect both of your methods to be void methods.)
What manipulation that gets implemented is entirely up to you: Increasing blue while decreasing red, flipping vertically, posterizing, etc. You must implement something NOT already implemented in the class Picture. You can use the methods that are there as examples, but do not make any calls to those existing methods to implement your new methods.
Turn in Picture.java file via T-Square. Name your methods something that makes sense for what it does and place those names in the top comments region of the Picture.java class definition. Place your methods at the bottom of the class definition, with a comment before it indicating what your method does.
Priscilla will compile your Picture.java file in DrJava and test it using the interactions pane. She will create an instance of a Picture, execute each of your defined methods on that Picture object and show() the results.
WHAT TO TURN IN
- Modified Picture.java.
- Please include your name in the beginning comments
- Picture.java should contain 2 new methods, one method using a for loop, the other using a while loop with comments explaining what each does.
Grading Criteria
- Compiles with no errors: 70 points
- Has a working Picture method using FOR loop: 10 points
- Has a working Picture method using WHILE loop: 10 points
- Good comments on each: 10 points
Homework 2
Modify Picture.java so that when run (i.e., running the main() method), a collage is generated and shown (via .show). Here are the specs that you have to meet:
- You must create a canvas picture of 1024 x 768. All of your pictures must be composed into the canvas.
- The same picture must appear at least four times: Once in its original form, then three more times modified in some way.
- You must use scale at least twice.
- You must use one other manipulation method in Picture (e.g., negate or oilPaint or flip).
- You must use a turtle do drop() a picture at least once.
- You must create a new Picture manipulation method, and use that in your collage as well.
Submit Picture.java as your turn-in. You must use good commenting style. You are bound by the honor code not to share code with anyone.
Grading Rubric
- 40 points: Compiles (10), runs (from main method) (20), and generates a picture of 1024x768 (10).
- 10 points: Same picture appears 4 times.
- 10 points: Scale is used twice.
- 10 points: Use at least one other Picture method.
- 10 points: Uses a turtle with drop()
- 20 points: Create and use a new picture method.
Homework 3: Write your name with turtle: Letter Turtle
You will need: LetterTurtle.java
For this assignment, you will be required to draw your name (at least four letters) using a LetterTurtle.
The Initials
You will be expected to draw the first four letters of your first name (if your first name is less than four letters, write your first name and the first initial of your last name). For me, Mark Guzdia, this means that I should draw the letters “MARK” (upper or lowercase) to receive credit.
The Shape of the Letters
We realize the difficulty of creating letters that have curves such as D, O, S, B to name a few. Those with initials that have curves may draw the “block” or “square” versions of the curved letters instead. Those wishing to attempt curved letters are welcome to do so for extra credit. Please see the extra credit section.
Extra Credit (+5 for each extra method)
In addition to the block form of the letters, you can choose 1 or 2 letters and write methods for the curved version. If there is no curved version of your initials, feel free to choose any curved letter to do. Thus for my initials “MARK,” I would have to write one method for the block version of “R” and a method for "M" and "A" and "K" to satisfy the minimum requirements. To receive extra credit, I could write a method for the curved version of “R”
Method Header
You will need to write at least a separate method for each letter. You should input the maximum height and width, and the letter should not be larger than that. The method names need to be appropriate so as to be self-documenting. The minimum requirement will be for both methods to be void, but non-void method will be accepted as well.
For example, the methods I would have to write would be something akin to the following:
public void drawM(int maxHeight, int maxWidth) {
//Method body in here
}
public void drawA(int maxHeight, int maxWidth)){
//Method body in here
}
public void drawK(int maxHeight, int maxWidth)){
//Method body in here
}
public void drawR(int maxHeight, int maxWidth)){
//Method body in here
}
Method Body
You will be required to do your drawing with the LetterTurtle on a World. To make the drawing easier to see, you need to change the Pen width of the LetterTurtle to something other than the default width of 1. The final resulting drawing cannot contain a visible LetterTurtle.
Useful Mathematical Values and Methods
When drawing your initials especially the curved ones, you will probably need some typical mathematical values and methods. You will find these useful values and methods within the Math class in the Java API:
http://java.sun.com/j2se/1.5.0/docs/api/java/lang/Math.html
For instance if I wanted to use the cos method and constant value PI, I could do something like the following:
double value = Math.cos(Math.PI * 0);
Casting
Because a great deal of the LetterTurtle movement methods require int values as inputs and most Math methods return double values, it is necessary to cast. In general, one of the java compiler’s tasks is to make sure that data types match across an assignment statement and between the formal and actual parameters of a method, and to show a compilation error if these are not consistent.
When we cast a value to a different data type, we inform the compiler that we really do know what we are doing, and that it should not worry about type consistency. Specifically, if we cast a double to type int, we are promising two things to the compiler:
1. We believe the contents of that double result to be small enough to fit in an int, and
2. We are happy to discard the fractional part of the double that is not stored in an int.
Here is the same line of code previously given:
double value = Math.cos(Math.PI * 0);
What if value was actually an int like so:
int value = Math.cos(Math.PI * 0);
Just looking at the code, you know you would get an error, because the types on either side of equal sign do not match. Currently we have:
int = double
We need the same type on both sides to get past the compiler. Because we need int for our LetterTurtle method, we are going to cast the right side of the equal sign to int:
int value = (int)(Math.cos(Math.PI * 0));
Here we finally have the same type on both sides:
int = int
It is important to note that to change a double to an int, the double is truncated at the decimal point. Essentially this means the decimal and everything to the right of it is thrown away. It is very important to note that does casting will not round the value up or down.
Examples (done in Dr. Java’s interactions pane):
> (int)5.123
5
> (int)6.523
6
> (int)-9.791
-9
Commenting
Though your method names will be self-documenting you should still comment your code. Place above each of your method names, comments about what the method will do. Also always place comments that include your name, email ID (gtx000x or jsmith3) and grading TA at the top of all the java files you submit.
Main Method
You should also include a main method within LetterTurtle to generate your name. You should move the turtle between each letter's drawing to space out the letters appropriately.
So putting it all together, my code would start to look like this:
import java.awt.*;
/*** Name:
*Prism ID(gtx000x or jsmith3):* Grading TA:
**/
public class LetterTurtle extends Turtle{
public LetterTurtle(World w){
super(w);
}
public void drawM(int maxHeight, int maxWidth) {
//Method body in here
}
public void drawA(int maxHeight, int maxWidth)){
//Method body in here
}
public void drawK(int maxHeight, int maxWidth)){
//Method body in here
}
public void drawR(int maxHeight, int maxWidth)){
//Method body in here
}
public static void main(String[] args){
World w = new World();
LetterTurtle me = new LetterTurtle(w);
// Position turtle here for first letter
me.drawM(100,100);
// Position turtle here for second letter
me.drawA(100,100);
}
}
What to Turn In
How to Turn In
Grading Rubric
- Code compiles without warnings or errors. 20 points.
- Opens a world and puts a turtle in it. 10 points.
- Creates methods for name letters. 30 points.
- Draws each letter on the World. 10 points.
- Spaces out the letters so that they are visible. 20 points.
- Stays within the maximum height and width. 10 points.
Homework 4: Use Weaving and Repeating to Create Music (Woven Music)
Using the methods developed in class to play with linked list of music, create a song.
- You must use weave and repeatNext (or repeatNextInserting) to create patterns in your music. (Which means, of course, that you have to use the SongNode and SongPhrase classes.)
- You must use weave and either of the repeats AT LEAST a TOTAL of FOUR TIMES in your piece. In other words, repeat a set of nodes, then repeat another set, then repeat another set. Then weave in nodes with one pattern, then weave in nodes with another pattern. That would be five. Or do one Repeat to create a basic tempo, then four weaves to bring in other motifs.
- You must use at least four unique riffs from SongPhrase. (It's okay for you to make all four of them yourself.)
- You must also create your own riffs in SongPhrase. You can simply type in music, or you can compute your riff – any way you want to do it is fine. Just create at least one phrase (of more than a couple notes) that is interesting and unique. That is, you must create at least one new method in SongPhrase and use it.
- All told, you must have at least 20 nodes in your final song.
You only have to use SongNode and have a single part with a single instrument. It does not need to autoplay!
You will create a class (with some cunning name like MyWovenSong) with a main that will assemble your song, then open it with showFromMeOn (or show–whatever you need to do open up the notation View on your masterpiece). Please put comments in your class:
- With your name, GT-email address
- Explaining what you're doing
Turn in to T-Square:
- Your song class.
- Your modified SongPhrase class.
- If you end up modifying SongNode or SongPart, you can include that, too.
- KEY: Draw a picture of your resultant list structure. Show us where all the nodes are in your final composition. You can do this by drawing with a tool like Paint or Visio or even PowerPoint, or you can draw it on paper then scan it in. You can turn in JPEG, TIFF, or PPT files.
Please consider sharing your song (by saving out the MIDI) in the Gallery HW4: Woven Music-Spring12
Grading Rubric
- Compiles: 10
- When run, generates a score: 10
- The linked list generating the score uses at least 20 SongNodes: 15
- The list is assembled from at least five repeats and weaves: 20 (4 each)
- There is a new method in SongPhrase that is used in the piece: 10
- There is a picture of the list structure: 10
- The picture is correct and reflects the nodes in the final composition: 10
- Code is clear and well-commented. 5
Homework 5: Use Weaving and Repeating to Create A Pattern (Woven Images)
Using the methods developed in class to create a pattern of repeated images with PositionedSceneElement. You are going to write code like this:
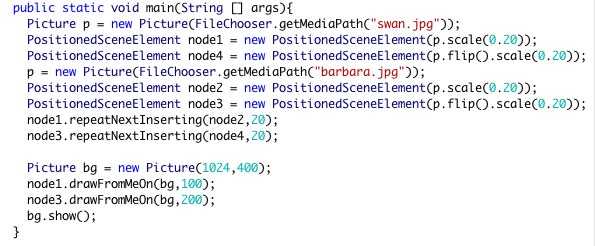
To produce images like this:
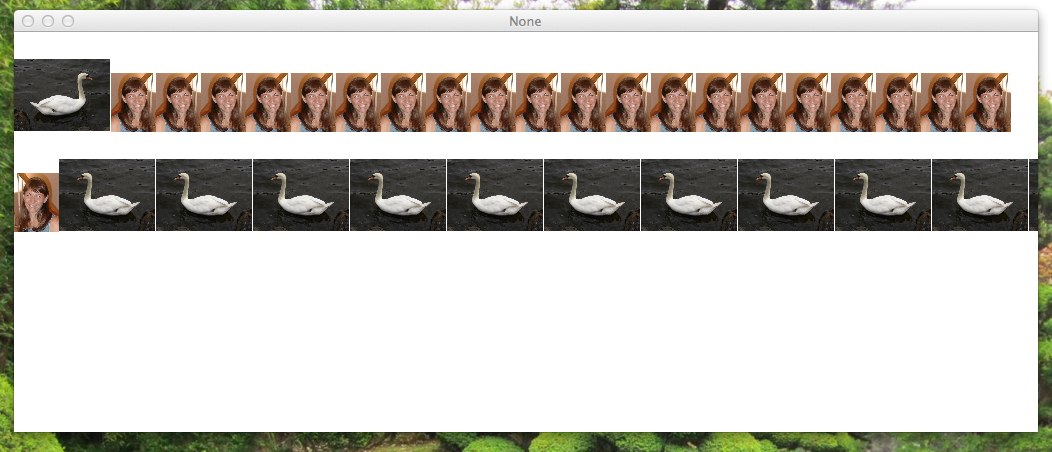
- You will IMPLEMENT drawFromMeOn(Picture canvas, int currentY) that draws the linked list starting with this on the canvas on the specified row.
- You will also IMPLEMENT weave and repeatNext and repeatNextInserting, and use these three to create patterns of images in a linked list.
- You must use weave and either of the repeats AT LEAST a TOTAL of FOUR TIMES in EACH LINE your composition. In other words, repeat a set of nodes, then repeat another set. Then weave in nodes with one pattern, then weave in nodes with another pattern. That would be four. Or do one Repeat to create a basic background, then three weaves to bring in other patterns.
- You are to create a background and weave onto FOUR LINES. (The example above has two lines.) That means that you will create four linked lists, then display all of them on a background.
- You will do this all in a main() method in PositionedSceneElement which creates the linked lists, displays them on a canvas, and then then shows the canvas.
You will be modifying PositionedSceneElement. Please put comments in your methods:
- With your name, GT-email address
- Explaining what you're doing
Turn in to T-Square:
- Your PositionedSceneElement class.
- Whatever pictures you use.
- KEY: Draw a picture of all FOUR of your resultant list structures. Show us where all the nodes are in your final composition. You can do this by drawing with a tool like Paint or Visio or even PowerPoint, or you can draw it on paper then scan it in. You can turn in JPEG, TIFF, or PPT files.
Please consider sharing your image (by saving out the picture as a JPEG or PNG) in the Gallery HW5: Woven Images-Spring12
Grading Rubric
- Compiles: 10
- When run, generates an image: 10
- There are four lines of images: 10
- Each list is assembled from at least four repeats and weaves: 32 (4 for each of 4 lines, so 16 total – two points each)
- There is a picture of the four list structures: 20 (5 points each)
- The picture is correct and reflects the nodes in the final composition: 10
- Code is clear and well-commented. 8
HW6: Doubly Linked Lists
For this homework, you are going to create a subclass of LayeredSceneElement called LayeredSceneElementDoubly which adds in previous links. LayeredSceneElementDoubly nodes should do everything that LayeredSceneElement nodes can do, but internally, they have a link to the previous node. See page 154 in the book for some discussion of doubly linked lists, and see the video http://www.cc.gatech.edu/~mark.guzdial/videos/sceneElementWithPrevious/ for an implementation of doubly linked lists with PositionedSceneElement.
Criteria that you must meet:
- Your new class must be able to respond to getPrevious() and setPrevious(), though setPrevious can be private.
- Your new class must implement remove correctly, which we do not do in the video.
- Your new class must implement both insertAfter (which is already in LayeredSceneElement) and insertBefore
- Your new class must implement both last() to find the last node in the list and first() to find the first node in the list.
- Your new class must implement drawFromMeBackward(bgPicture) which traverses the list from the given node to the FRONT of the list, drawing onto the bgPicture.
- Your new class must keep all the next and previous links correctly organized, e.g., for any node except the last, node == node.getNext().getPrevious().
- Because you are creating a subclass, rather than a new class (as in the video), your subclass will not have to recreate all methods from the superclass. Only include the methods in the subclass that will necessarily be different than in the superclass.
You will implement your LayeredSceneElementDoubly class with a PARTNER using PAIRED PROGRAMMING. THIS IS A REQUIREMENT. Any solo efforts will lose 15 points immediately. You can learn more about Pair Programming. In your comments, you must state who your partner is and state, "No more than 25% of the program code was written apart from one another. Each of us spent half of the time together at the keyboard, with the other one watching over and navigating."
Test code
Here's how we might test your code, and what the points will be worth. (I'm writing this freestyle, so beware of typos below. If there is casting to be done to make it work, we can fit that in.)
Picture ptree = new Picture(FileChooser.getMediaPath("tree-blue.jpg"));
Picture pdog = new Picture(FileChooser.getMediaPath("tree-dog.jpg"));
LayeredSceneElementDoubly node1 = new LayeredSceneElementDoubly(ptree,10,10); // 10 points for getting the class to work
node1.insertAfter(new LayeredSceneElementDoubly(pdog,20,10));
node1.getNext().insertBefore(new LayeredSceneElementDoubly(pdog.scale(0.5),30,10);
System.out.println(node1.getNext().getNext() == node1.last()); // Should be true. 5 points
System.out.println(node1.getNext() == node1.getNext().getNext().getPrevious()); // Should be true. 10 points for getting the links correct here
System.out.println(node1 == node1.getNext().first()); // Should be true. 5 points
Picture bg = new Picture(500,500);
node1.drawFromMeOn(bg); // Should work. 10 points
Picture bg2 = new Picture(500,500);
node1.last().drawFromMeBackwards(bg2); // Should work. 20 points.
node1.remove(node1.getNext()); // 20 points
// 20 points for not implementing anything in the subclass that could just be inherited from superclass.
Here's what the code might look like with all the correct casts
public class TestCode2 {
public static void main (String [] args){
Picture ptree = new Picture(FileChooser.getMediaPath("tree-blue.jpg"));
Picture pdog = new Picture(FileChooser.getMediaPath("tree-dog.jpg"));
LayeredSceneElementDoubly node1 = new LayeredSceneElementDoubly(ptree,10,10); // 10 points for getting the class to work
node1.insertAfter(new LayeredSceneElementDoubly(pdog,20,10));
((LayeredSceneElementDoubly) node1.getNext()).insertBefore(new LayeredSceneElementDoubly(pdog.scale(0.5),30,10));
System.out.println(node1.getNext().getNext() == node1.last()); // Should be true. 5 points
System.out.println(node1.getNext() == ((LayeredSceneElementDoubly)node1.getNext().getNext()).getPrevious()); // Should be true. 10 points for getting the links correct here
System.out.println(node1 == ((LayeredSceneElementDoubly)node1.getNext()).first()); // Should be true. 5 points
Picture bg = new Picture(500,500);
node1.drawFromMeOn(bg); // Should work. 10 points
Picture bg2 = new Picture(500,500);
((LayeredSceneElementDoubly)node1.last()).drawFromMeBackward(bg2); // Should work. 20 points.
node1.remove(node1.getNext()); // 20 points
// 20 points for not implementing anything in the subclass that could just be inherited from superclass.
}
}
Homework 7: Make a Movie
Use the same classes as in WolfAttackMovie, but tell a different story with different pictures.
Criteria
- 25 points. Your tree must include at least 20 BlueScreenNode instances and at least 5 branches of some kind.
- 20 points. You must make all your own pictures: no tree-blue.jpg, no dog-blue.jpg, no house-blue.jpg, but you must use blue backgrounds.
- 5 points. At least one of your pictures should be text on a blue background. Make it a caption, or a speech bubble.
- 25 points. The movie should be at least 60 frames long.
- 10 points. You must remove at least one branch during the movie, and insert one branch during the movie.
- 15 points. Make it make sense – interesting, maybe even have a plot.
Turn in your animation class and all your JPEG files.
You MUST do this as a Pair Programming activity, or you lose 15 points. Include an honor statement with your partner's name (both of you turn in the same code, and collaborate with no one else but your partner) and a claim that you each typed in approximately half of the overall code.
Not a requirement: Please do generate an AVI or MOV and uploading it here: HW7: Digital movies you created - Spring 2012
Homework 8: Sprite Animation
Two parts to this assignment:
(65 points) A. Create a class SpriteAnimation that will hold a list of pictures (using any data structure you choose) and a pointer to the current picture. A SpriteAnimation understands an add() method that takes another picture and adds it to the list of pictures. Each time a SpriteAnimation is told to getNext(), it returns the picture currently pointed to by the current-picture-pointer, then moves the current-picture-pointer to the next picture. When the current-picture-pointer gets to the end of the list, it moves to the start of the list. (20 points for creating the class with the right instance variables, 10 points for a working constructor, 10 points for a working add() method, 10 points for a working getNext() method that returns a picture, 15 points for getting the circularity right.
I'm happy for you to define getNext() in other ways, but here's what needs to happen:
- I add Picture1 to an instance of SpriteAnimation
- I add Picture2
- When I call getNext() on the instance of SpriteAnimation, I get Picture1
- When I call getNext() again, I get Picture2
- When I call getNext() again, I get Picture1
(35 points) B. Create a main() method in SpriteAnimation that creates a sprite animation and fills it with at least four pictures. Generate an animation (using FrameSequence) with at least 30 frames using any additional pictures you want and also using your SpriteAnimation instance. (10 points for creating a SpriteAnimation, 5 points for filling it correctly with pictures, 10 points for generating an animation using FrameSequence, and 10 points for making it at least 30 frames long.)
Bonus 10 points: Make your animation move around the frame.
You must do this assignment in a pair.
Homework 9: Add the Hunters
Modify the Foxes and Rabbits Greenfoot scenario (like how we did in the adding Corn video) to add a Hunter class.
- The Hunter doesn't get old or get hungry or breed.
- He can see up to three cells away.
- He can shoot (kill, consume as if food) up to two foxes per turn.
There are three parts to this assignment:
- Implement the Hunter as described. Create three hunters and drop them into the Foxes and Rabbits simulation. Call this simulation "foxes-and-rabbits-and-hunters."
- If you already have a pretty good equilibrium with Part #1, you can skip Part #2 and move on to Part #3. Now, save a copy of your simulation. Create another version of this simulation, where you try to maintain more equilibrium. You are welcome to change any one variable in your simulation, such as decreasing the number of hunters, decreasing how far away they can see, increasing the fox likelihood percentage in Field (thus creating more foxes), increasing the breeding variables for foxes. Call this simulation "foxes-and-rabbits-and-hunters-balanced." In your comments in the class Hunter, explain what you changed and why you think it worked.
- Now make another copy of "foxes-and-rabbits-and-hunters." Give hunters the ability to shoot up to one fox or rabbit per turn (only one shot object per call to act()). Try to find a setting of number of hunters and variables on foxes and rabbits where this new ecology is balanced. Call this simulation "foxes-and-rabbits-and-omnivore-hunters". In your comments in the class Hunter, explain what you changed and why you think it worked.
Turn in a zip file containing all three scenarios.
Grading Rubric
- 20 points for creating the Hunter class and placing 3 hunters in the world.
- 20 points for successfully seeing up to three cells away and killing up to two foxes per turn.
- 10 points for creating a new version that is more stable.
- 10 points for clear and reasonable comments about why the new version is more stable.
- 20 points for creating a Hunter that can kill a fox or a rabbit (up to 3 cells away).
- 10 points for creating an Omnivore Hunter world that is (relatively) stable.
- 10 points for clear and reasonable comments about how you achieved stability.
You must do this as a pair (or triplet) or receive -15.
Homework 10: Disease Propagation and Public Policy
Do Exercise 16.10 on Page 430 in the book. Make sure that you do 3 runs of the initial condition, so that you can compare the health policies to the baseline.
Grading Rubric
- 30 points: Successfully implement the variation of the disease propagation simulation described: Typhoid Mary (10 points), Healthy people who turn away (10 points), and 25% death rate and infection states (10 points).
- 40 Implement two public health policies (20 points each)
- 10 Initial report (3 runs with graphs)
- 20 Reports on the two health policies (10 points each) including three runs with graphs, and a text explanation of how it worked.
Hand in your code, and also your report (as Word or PDF)
You must do this as a pair (or triplet, if necessary), or receive -15.
OPTIONAL Homework 11: Villagers with Images
This is an optional homework, for extra credit only. Any points on this go toward your homework grade.
Do Exercise 16.6 on page 429. Turn in a zip file with the classes you add to the Simulation classes (and any Simulation classes you changed), as well as the JPEG images that you use to represent the villagers and the NBD. (Each pair turns in the same zip file.)
Grading Rubric
- 5 points: Create a subclass of the simulation called CrowdSimulation that runs.
- 5 points: Create 100 villagers in this world.
- 5 points: Quality of Villagers appearance.
- 10 points: Villagers try to move together.
- 10 points: Villagers avoid too much crowd.
- 20 points: Villagers mill about changing their direction and waving.
- 10 points: NBD enters at timestep 10, and walks across the scene slowly.
- 5 points: Quality of NBD's appearance. (5 Extra Points if NBD changes appearance while walking!)
- 20 points: Villagers notice NBD and move away if NBD is close.
- 10 points: If NBD is NOT close, they simply follow all the other rules.
There is an additional 20 points available! Implement the villagers and NBD as a Sprite Simulation (10 points each), using the class you created for HW8!