Study Guide Midterm 2 Spring 2011
Study Guide for Midterm REMOVED
Q1. Duplicate the List
Below is the code for weave() which makes count copies of the node nextOne inserts them into the list that starts at this every skipAmount nodes.
/**
*Weave the input sound count times every skipAmount elements* @param nextOne SoundElement to be copied into the list
*@param count how many times to copy
* @param skipAmount how many nodes to skip per weave
*/
public void weave(SoundElement nextOne, int count, int skipAmount)
{
SoundElement current = this; // Start from here
SoundElement copy; // Where we keep the one to be weaved in
SoundElement oldNext; // Need this to insert properly
int skipped; // Number skipped currently
for (int i=1; i <= count; i++)
{
copy = nextOne.copyNode(); // Make a copy
//Skip skipAmount nodes
skipped = 1;
while ((current.getNext() != null) && (skipped <= skipAmount))
{
current = current.getNext();
skipped++;
};
if (current.getNext() == null) // Did we actually get to the end early?
break; // Leave the loop
oldNext = current.getNext(); // Save its next
current.insertAfter(copy); // Insert the copy after this one
current = oldNext; // Continue on with the rest
}
}
Write a new method that copies a whole list, start from this. Each node in the this list should be copied and connected up to a copy of this, in the same order. The method copyList returns the copy of the node this. If a list looks like A-B-C, this method should return (copy of A)-(copy of B)-(copy of C)
public SoundElement copyList(){
}
You can share answers at Q1: Study Guide Midterm 2 Spring 2011
Q2. Imagine that you have a collection of nodes that have links like the below. Let’s say that node1 is pointing to an instance of class PictureNodeBranch and that all other nodes are instances of class PictureNodeDoubly:
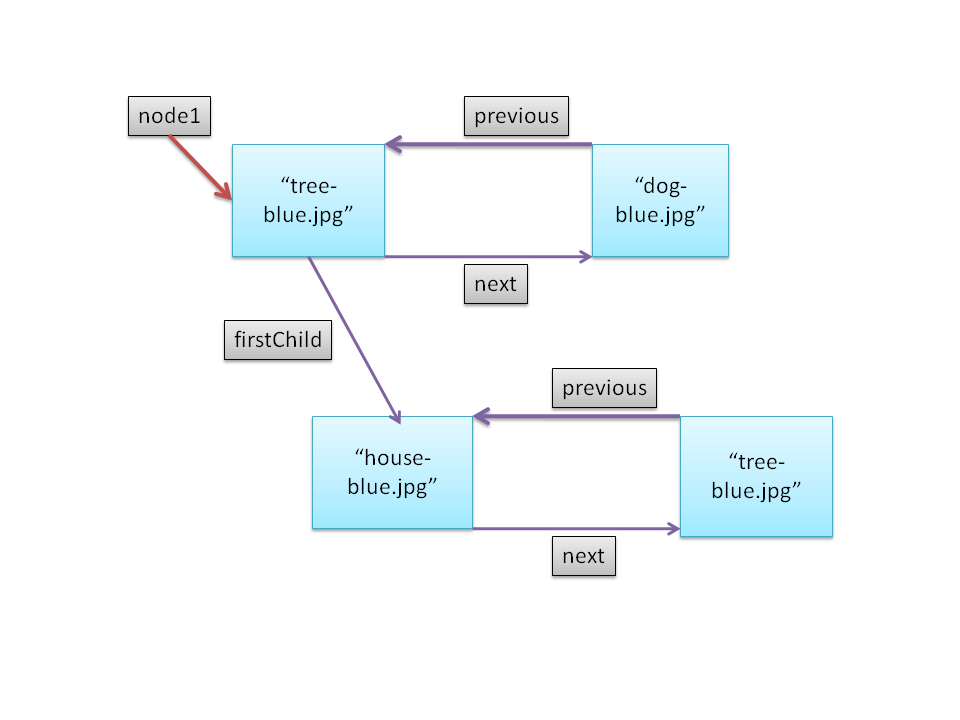
You should assume that the accessors and manipulators getPrevious, getNext, setPrevious, setNext, and getFirstChild and setFirstChild all exist. You may not use any other methods (e.g., remove, add, last, insertAfter).
a. Imagine that you have a new node referenced by variable newNode (containing, say, “monster.jpg”). What statements would you use to insert newNode between the nodes containing “house-blue.jpg” and “tree-blue.jpg”? You only have the variable reference to node1 to start from, but you can assume that you know the structure above.
b. Now remove the node from the list containing "house-blue.jpg" and make sure that the firstChild , next, and previous links are correct.
You can share answers at Q2: Study Guide Midterm 2 Spring 2011
Q3. Review the class structure for the WolfAttackMovie.
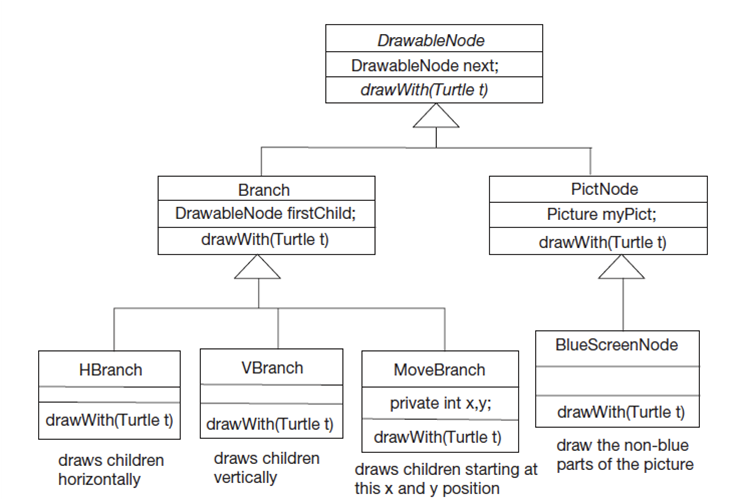
a. What are all the methods and fields (instance variables) known to a BlueScreenNode?
b. What does an instance of BlueScreenNode know or have that a PictNode doesn't?
c. An HBranch instance has a firstChild link. Why? Where did it come from?
d. Here is a method for HBranch.
/**
* Ask all our children to draw,
* then tell the next element to draw
* @param turtle Turtle to draw with
*/
public void drawWith(Turtle turtle) {
// start with the first child
DrawableNode current = this.getFirstChild();
// Have my children draw
while (current != null) {
current.drawWith(turtle);
turtle.moveTo(turtle.getXPos()+gap,turtle.getYPos());
current = current.getNext();
}
// Have my next draw
if (this.getNext() != null) {
current = this.getNext();
current.drawWith(turtle);
}
}
}
For each field and method above that is NOT defined in HBranch, tell me which class it IS defined in.
You can share answers at Q3: Study Guide Midterm 2 Spring 2011
Link to this Page